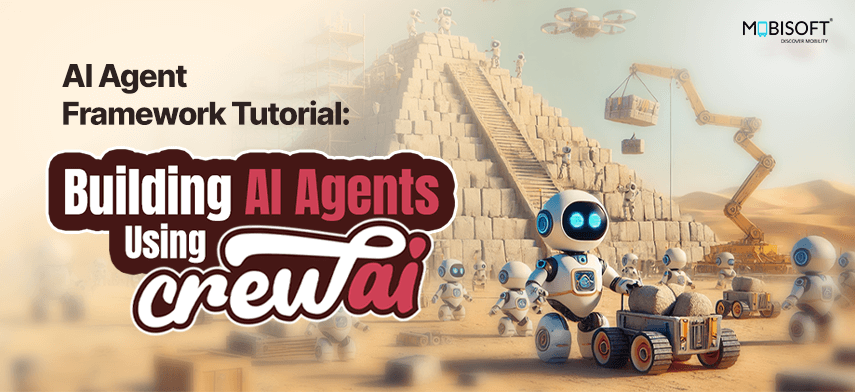
In today’s tech landscape, building AI agents are at the forefront of innovation, transforming how we approach automation and intelligent systems. In this tutorial, we’ll explore how to build AI agents using CrewAI, a powerful AI agent framework renowned for its advanced engine capabilities, including flexible rule application and robust memory features.
CrewAI offers a powerful CLI that generates a comprehensive project structure, allowing for AI agent development with prompts written in YAML files. However, for newcomers, this complexity can be overwhelming. That’s why we’re starting with a straightforward example: creating a fully functional AI agent within a single Python file. Later, we’ll dive deeper into leveraging the CrewAI CLI for building more complex projects.
Key Concepts in Building AI Agents: Tasks and Agents
A fundamental concept in the CrewAI framework is the distinction between tasks and agents. When building AI agents, you’re essentially orchestrating a set of agents, each assigned to execute specific tasks.
Let’s say we want to create an AI agent that writes blog posts for us. (Yes, yet another blog post written by an AI agent!). Let’s assume we do it in 2 steps. Given a blog title, the first agent creates an outline of the blog, and the second agent takes the outline as input and writes a full blog post by strictly following that outline.
So here we have 2 agents, each of which performs 1 task:
- Agent: Blog Outline Generator
Task: Generate Blog Outline - Agent: Blog Writer
Task: Write Blog
Let’s see how we can do this in code!
Step 1: Setting Up Your AI Agent Framework Project
CrewAI needs Python 3.10 or higher.
Run `pip install crewai` to ensure it’s available. Also, run `pip install python-dotenv` to ensure that you have the dotenv package installed.
Create a directory for your project.
mkdir crewaidemo
cd crewaidemo
Create a .env file and add the following content in it.
MODEL=gpt-4o-mini
OPENAI_API_KEY=<Your-Open-AI-key>
Code language: HTML, XML (xml)
Here we are specifying that we are going to use the ‘gpt-4o-mini’ model from Open AI. To use that model, we will need to provide an OpenAI API key.
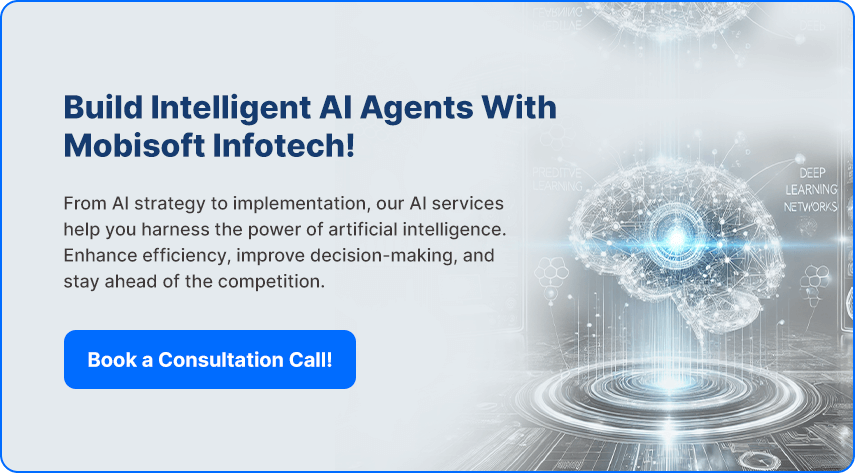
Step 2: Writing Code to Build AI Agents
Create a file main.py
and add the following code to it.
from crewai import Agent, Crew, Process, Task
from dotenv import load_dotenv
# Load environment variables from .env file
load_dotenv()
# Enable debug logging for LLM calls
import litellm
litellm._turn_on_debug()
blog_outline_generator = Agent(
role="Blog Outline Generator",
goal="Given a title: {blog_title}, generate a short blog outline which is only 100 words and only 5 subheadings which will be used to write a blog post with 2000 words",
backstory="You are a blog outline generator"
)
generate_blog_outline_task = Task(
description="Given a title: {blog_title}, generate a short blog outline which is only 100 words and only 5 subheadings which will be used to write a blog post with 2000 words",
expected_output="A blog outline",
agent=blog_outline_generator,
)
blog_writer = Agent(
role="Blog Writer",
goal="Given a blog outline for the title: {blog_title}, write a blog post with 2000 words",
backstory="You are a blog writer"
)
write_blog_task = Task(
description="Given a blog outline for the title: {blog_title}, write a blog post with 2000 words",
expected_output="A blog post, make sure to include main points from blog outline which you have received in the context in the blog post as subheadings",
agent=blog_writer,
context=[generate_blog_outline_task]
)
crew = Crew(
agents=[blog_outline_generator, blog_writer],
tasks=[generate_blog_outline_task, write_blog_task],
process=Process.sequential,
verbose=True
)
result = crew.kickoff(inputs={'blog_title': "Violence in movies"})
print(result)
Code language: PHP (php)
That’s it. In less than 40 lines of Python code, you have your first working AI agent!
Don’t worry if it doesn’t make a lot of sense. I have explained the code below section by section.
If you want to try it out, just run:
python main.py
Code language: CSS (css)
It will print a lot of debugging output, and at the end, it should print your blog post! Something like the following:
# Violence in Movies: A Deep Dive into Its Evolution, Impact, and Future
Violence has long been a captivating element within cinema, serving as a veritable tapestry woven through the various genres and eras of filmmaking. As we navigate through the numerous layers surrounding this topic, we will explore the historical context from which depictions of violence in film have emerged, the psychological ramifications for audiences, the role of censorship, cultural reflections, and finally, a glimpse into the future of violence in movies. Let's embark on this journey.
## The Historical Context of Violence in Film
...
...
Code language: PHP (php)
Step 3: Understanding Code Execution for AI Agent Frameworks
Let’s break down the key sections of the code so you can better understand how the AI agent architecture works in CrewAI.
from crewai import Agent, Crew, Process, Task
from dotenv import load_dotenv
# Load environment variables from .env file
load_dotenv()
# Enable debug logging for LLM calls
import litellm
litellm._turn_on_debug()
Code language: CSS (css)
Here, we have imported the Agent, Crew, Process, and Task classes from the CrewAI
package. Then, we load the .env
file with the load_dotenv
function to set up the environment for AI-driven automation.
Also, note that we’ve imported the litellm
package, which CrewAI
uses to make the actual LLM API calls. We’re enabling its debug output to help new agent creators understand the flow behind the scenes. If you find this output too distracting, you can comment out those lines!
Now, let’s create our blog_outline_generator agent.
blog_outline_generator = Agent(
role="Blog Outline Generator",
goal="Given a title: {blog_title}, generate a short blog outline which is only 100 words and only 5 subheadings which will be used to write a blog post with 2000 words",
backstory="You are a blog outline generator"
)
Code language: JavaScript (javascript)
In this section, we create an instance of the Agent
class named blog_outline_generator
. This agent has a specific role and goal: to generate a concise blog outline based on a provided title. Always write a meaningful role and a detailed goal. This will ensure that your agent works properly in the real world. The backstory gives context to the agent’s purpose, enhancing its functionality.
Here note the {blog_title}
. This is how we pass external user input to the agent. Later, while creating our Crew
object, we will pass the actual value for this parameter.
generate_blog_outline_task = Task(
description="Given a title: {blog_title}, generate a short blog outline which is only 100 words and only 5 subheadings which will be used to write a blog post with 2000 words",
expected_output="A blog outline",
agent=blog_outline_generator,
)
Code language: JavaScript (javascript)
Here, we define a Task called generate_blog_outline_task
. This task describes what needs to be done (generate a blog outline) and specifies the expected output. It is associated with the blog_outline_generator
agent, linking the task to the agent responsible for executing it.
blog_writer = Agent(
role="Blog Writer",
goal="Given a blog outline for the title: {blog_title}, write a blog post with 2000 words",
backstory="You are a blog writer"
)
Code language: JavaScript (javascript)
Next, we create another agent called blog_writer
. This AI-powered agent will expand the outline into a full blog post of 2000 words, effectively utilizing AI-driven automation.
write_blog_task = Task(
description="Given a blog outline for the title: {blog_title}, write a blog post with 2000 words",
expected_output="A blog post, make sure to include main points from blog outline which you have received in the context in the blog post as subheadings",
agent=blog_writer,
context=[generate_blog_outline_task]
)
Code language: JavaScript (javascript)
In this section, we define the write_blog_task
, which instructs the blog_writer
agent to create a blog post based on the outline provided. The task description specifies the expected output. Most importantly, it includes the context of the previous task, ensuring that the writer has access to the outline.
crew = Crew(
agents=[blog_outline_generator, blog_writer],
tasks=[generate_blog_outline_task, write_blog_task],
process=Process.sequential,
verbose=True
)
Code language: PHP (php)
Here, we create a Crew instance that brings together the agents and tasks we defined earlier. The process is set to sequential, meaning the tasks will be executed one after the other. CrewAI also supports another type of flow called ‘Hierarchical’ but that’s a subject of another blog post. The verbose flag is set to True, allowing for detailed logging during execution
result = crew.kickoff(inputs={'blog_title': "Violence in movies"})
print(result)
Code language: PHP (php)
Finally, we initiate the AI-driven automation process by calling the kickoff
method on the crew
instance, passing in a dictionary with the blog title as input. The result of the process is printed, which will include the final blog post.
Conclusion
That’s it! That’s how easy it is to create a working AI agent using CrewAI. I hope that I have managed to break down the process of creating AI agents in easy-to-understand steps. In the next post, we’ll dive deeper into how to use CrewAI
CLI to scaffold your agents and how to take a structured approach to build more complex AI agents.
Be sure to subscribe to our blog using the ‘Let’s Stay Connected’ form below!
If you’re looking to integrate AI agents into your workflow, AI strategy consulting can help you design an effective plan. Need expert guidance? Contact us to get started.
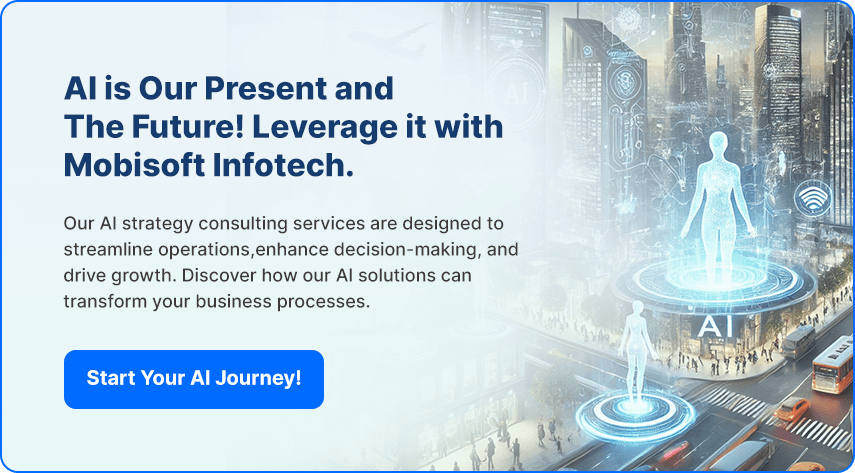
Author's Bio:
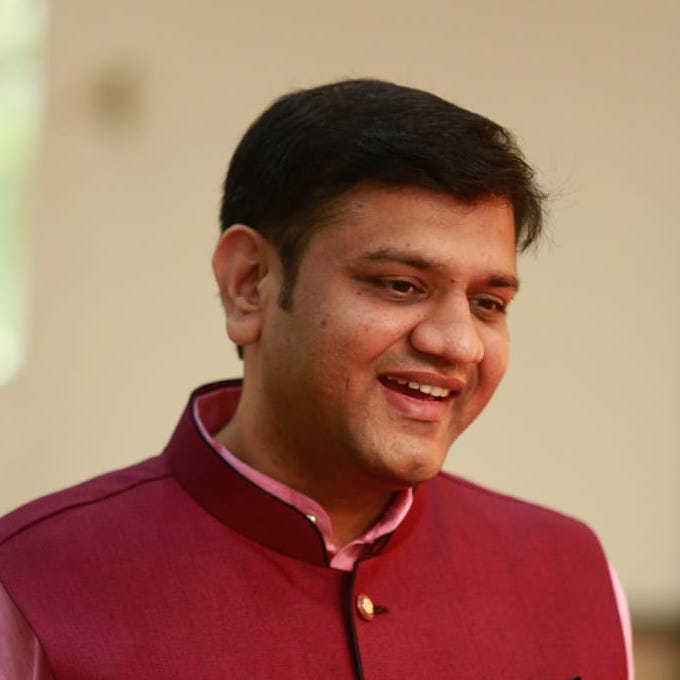
Pritam Barhate, with an experience of 14+ years in technology, heads Technology Innovation at Mobisoft Infotech. He has a rich experience in design and development. He has been a consultant for a variety of industries and startups. At Mobisoft Infotech, he primarily focuses on technology resources and develops the most advanced solutions.