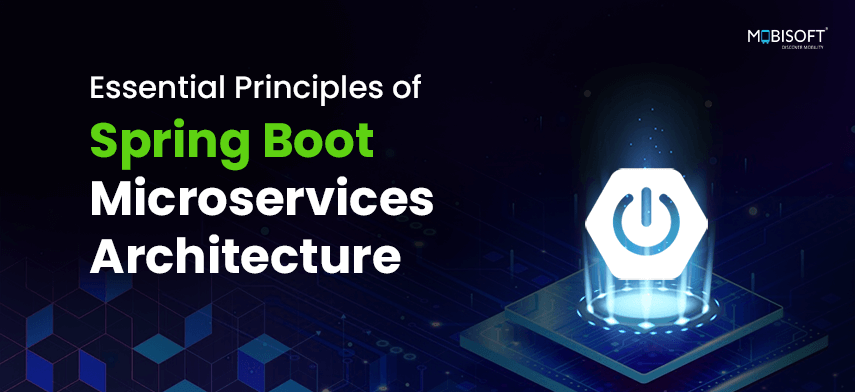
1. What are Microservices?
In today’s fast-paced digital world, applications need to be agile, scalable, and resilient. That’s where microservices come in. Think of them as small, independent building blocks that work together to create a larger application aviator game play demo. Each block focuses on a specific task, making the whole system more flexible and easier to manage. So, what exactly are microservices?
Simply put, they are small, independently deployable services that handle a specific business function. Imagine an online store: instead of one massive application, you might have separate microservices for managing user accounts, processing orders, handling payments, and tracking inventory. Each of these services can be developed, deployed, and scaled independently.
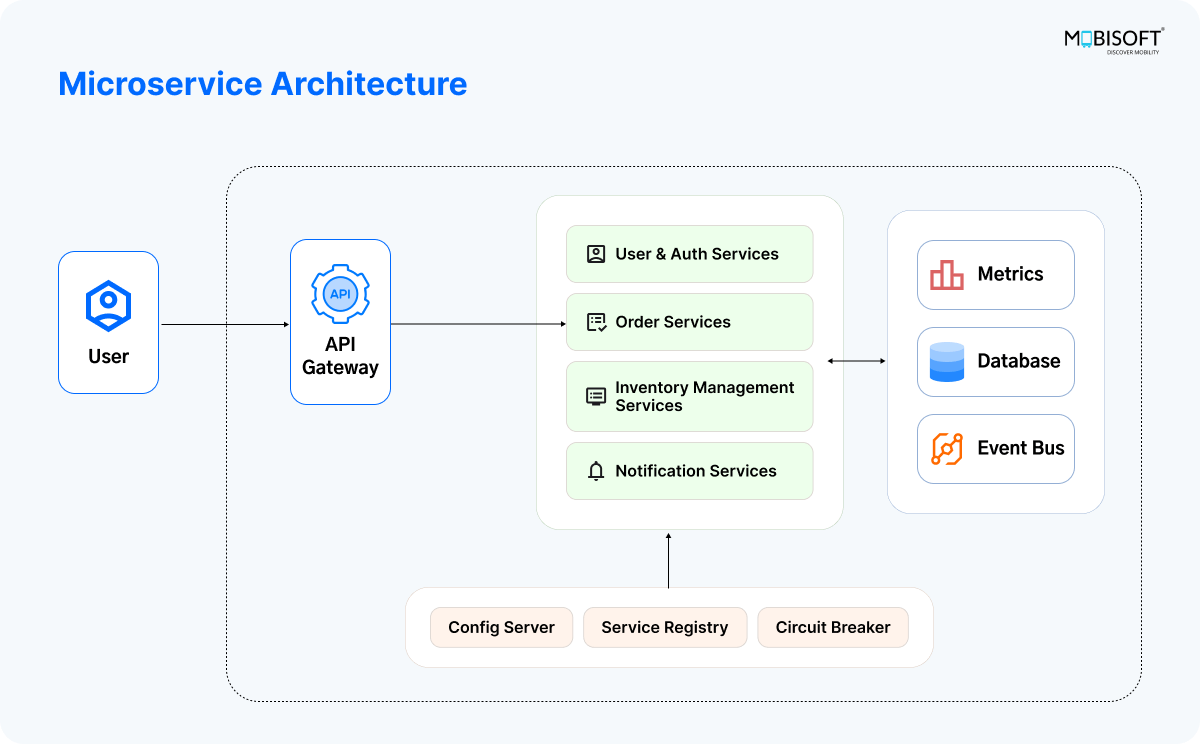
Characteristics That Define Microservices
- Single Responsibility: Each microservice has one job and does it well. It focuses on a specific business capability. For example, a “User Service” handles everything related to user accounts, while a “Product Service” manages product information. This clear separation makes each service easier to understand, build, and maintain.
- Loose Coupling: Microservices are designed to be independent of each other. This means changes to one service don’t require changes to others. If you update the “Product Service,” the “User Service” can continue running smoothly. This independence prevents cascading failures and simplifies deployments.
- Autonomy: Teams have the freedom to choose the best tools and technologies for each microservice. One service might be built with Python, while another uses Java or Node.js. This flexibility allows teams to use the right tool for the job and encourages innovation.
Why Are So Many Companies Adopting Microservices? Here Are Some Compelling Reasons:
- Scalability: Need more processing power for handling orders during a flash sale? You can scale just the “Order Processing” microservice without scaling the entire application. This efficient use of resources saves time and money.
- Faster Time to Market: Because teams can work on and deploy services independently, updates and new features can be released much faster. This agility gives businesses a competitive edge.
- Improved Fault Isolation: If one microservice fails, it doesn’t bring down the entire application. The other services can continue to function, minimizing disruption to users.
- Easier Maintenance: Smaller codebases are easier to understand, test, and update. This makes maintenance less complex and improves overall code quality.
In essence, microservices architecture offers a powerful approach to building complex applications. By breaking down applications into smaller, manageable pieces, businesses can achieve greater agility, scalability, and resilience.
2. Spring Boot
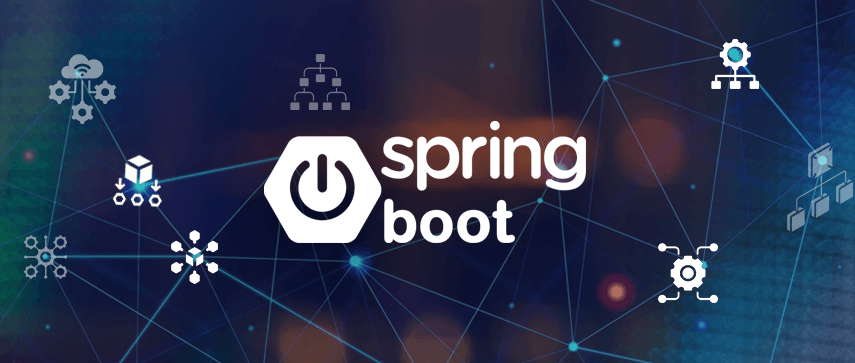
Building modern applications, especially microservices, can be complex. Setting up the necessary infrastructure and configurations can take a lot of time and effort, pulling developers away from what they do best: writing code. That’s where Spring Boot comes in.
Think of Spring Boot as a powerful toolkit that simplifies the development of Spring applications. It’s built on top of the popular Spring Framework but streamlines the entire process, making it much faster and easier to get started. Spring Boot is particularly well-suited for building microservices, providing a solid foundation for creating scalable and maintainable applications.
So, what makes Spring Boot so special? Here are some of its key features:
Auto-Configuration: This is a game-changer. Spring Boot automatically configures your Spring application based on the libraries you’ve included in your project. It intelligently figures out the necessary settings, reducing the need for manual configuration and saving you a ton of time.
Spring Boot Starters: These are pre-packaged sets of dependencies that make it incredibly easy to include common libraries in your project. For instance, if you’re building a web application, you can simply include the spring-boot-starter-web dependency, and Spring Boot will automatically include everything you need to get started, like Spring MVC, Tomcat, and Jackson for JSON processing. It’s like having a pre-assembled starter kit for different types of applications.
Embedded Servers: Spring Boot supports embedded servers like Tomcat, Jetty, and Undertow. This means you don’t need to install and configure a separate server. Your application can run independently, making deployment much simpler. You can just run it as a standalone JAR file.
Production-Ready Features: Spring Boot includes built-in support for essential production features like metrics (for monitoring application performance), health checks (for determining the status of your application), and externalized configuration (for managing settings outside of your code). These features make it easier to deploy and manage your applications in a real-world environment.
In short, Spring Boot takes away much of the boilerplate and complexity associated with building Spring applications. It lets developers focus on writing business logic and delivering value, rather than getting bogged down in configuration details. If you’re building microservices or any other type of Spring application, Spring Boot is definitely worth exploring.
3. Service Discovery
Service discovery is a critical component in modern microservices architectures, acting as the glue that keeps everything connected. Think of it like a dynamic phone book for your services – instead of hard-coding connections between different parts of your application, services can automatically find and talk to each other. This flexibility is particularly valuable in today’s cloud environments, where services often come and go as they scale up or down.
When it comes to implementing service discovery, there are several battle-tested tools at your disposal. Netflix’s Eureka has gained widespread adoption, offering a straightforward way for services to say “hey, I’m here!” and look up other services they need to communicate with. Another popular option is HashiCorp’s Consul, which goes beyond basic service discovery by also keeping an eye on service health and providing a distributed configuration store. Think of Consul as a Swiss Army knife for your microservices infrastructure – it not only helps services find each other but also ensures they’re healthy and properly configured.
4. API Gateway
The API Gateway acts as a single front door for all client requests. When a customer (client) wants to interact with any of your microservices, they go through the API Gateway. It then takes care of directing the request to the correct service, like a receptionist directing visitors to the right office.
Here’s what the API Gateway does:
Routing: It figures out which microservice should handle a particular request.
Composition: Sometimes, a single client request might require data from multiple microservices. The API Gateway can combine the responses from these services and send a single, unified response back to the client.
Protocol Translation: Different microservices might use different communication protocols. The API Gateway can translate between these protocols, ensuring seamless communication.
Using an API Gateway brings several key benefits:
- Centralized Authentication: Instead of each microservice handling its own security, the API Gateway can manage authentication and authorization for all of them. This simplifies security management and ensures consistency. It’s like having a security checkpoint at the mall entrance.
- Rate Limiting: The API Gateway can prevent any one client from overwhelming the backend services by limiting the number of requests they can make within a given time.
- Load Balancing: If you have multiple instances of a microservice running, the API Gateway can distribute incoming requests across them evenly.
In short, the API Gateway simplifies client interactions with your microservices architecture, improves security, and enhances performance. It’s an essential component for building robust and scalable microservices systems.
5. Configuration Management
Imagine you have many different applications (your microservices) running in various environments (development, testing, production). Each application needs its own set of settings, like database connections, API keys, and other parameters. Managing these settings individually for each application can quickly become a nightmare. That’s where configuration management comes in, offering a much better solution.
Think of it as having a central control panel for all your application settings. Instead of scattering configuration files across different servers and applications, you manage them in one central location. This approach offers several advantages, especially when dealing with many microservices:
- Centralized Configuration: Managing all configuration properties in one place makes it much easier to update settings and ensure consistency across all your services. If you need to change a database password, you only need to change it in one place, and all affected applications will automatically pick up the change. This simplifies operations and reduces the risk of errors.
- Spring Cloud Config: One popular tool for centralized configuration in a microservices environment is Spring Cloud Config. This acts as a dedicated configuration server. It stores your application settings in a version control system like Git (or other sources). This means you can track changes to your configurations, revert to previous versions if needed, and manage configurations for different environments (development, testing, production) separately. When an application starts, it connects to the Spring Cloud Config server to retrieve its settings. This ensures that every application has the correct configuration for its environment, simplifying deployments and promoting consistency.
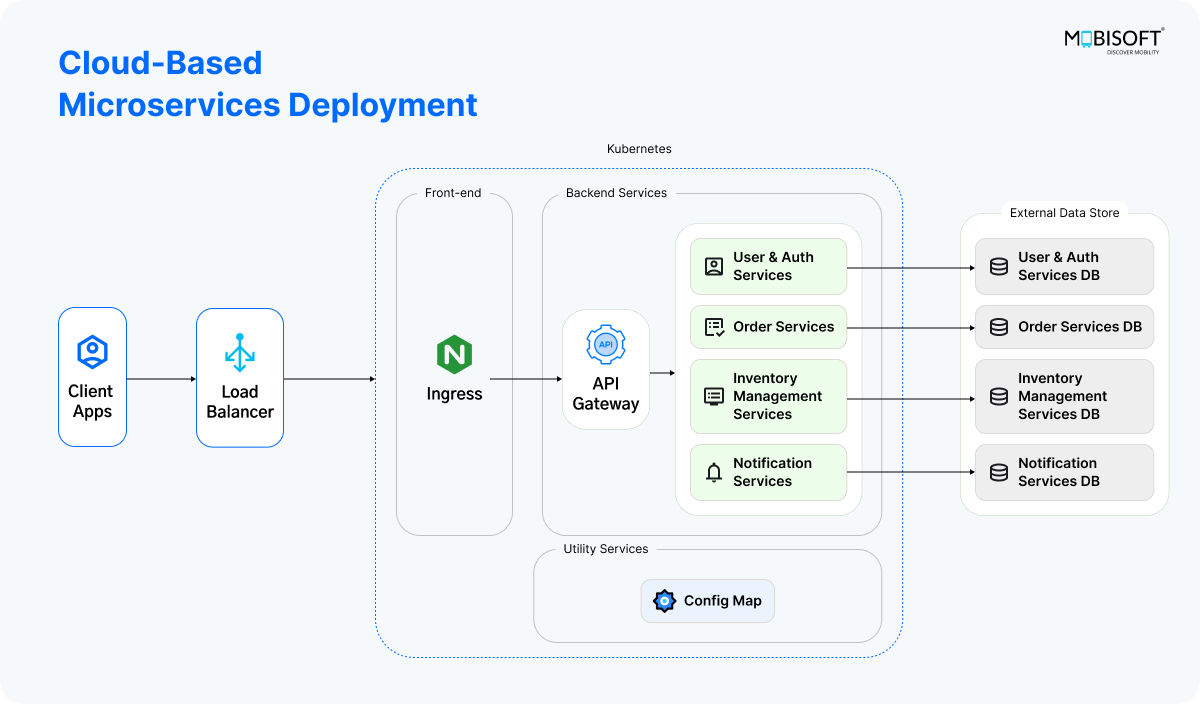
In essence, configuration management, especially with tools like Spring Cloud Config, provides a streamlined and robust way to manage application settings in a microservices architecture. It simplifies updates, ensures consistency, and makes it easier to manage configurations across different environments.
6. Circuit Breaker
Think of a circuit breaker in software like the one in your home’s electrical system. It’s there to stop problems from spreading. In software, a circuit breaker helps prevent one failing service from dragging down the rest of your system. If a service is likely to fail, the circuit breaker stops requests to it, giving everything a chance to stabilize and recover.
How Do You Use It?
There are some handy tools to help you implement circuit breakers:
- Resilience4j: This is a modern, lightweight library for Java. It helps build robust applications by offering features like circuit breakers, rate limiters, and more, ensuring your system can handle failures gracefully.
- Hystrix: Created by Netflix, Hystrix is another tool that implements the circuit breaker pattern. It allows you to set up fallback methods and keeps service calls isolated. Although it’s no longer actively developed, many people still find it useful.
A Simple Example: Imagine your Order Service goes down. Without a circuit breaker, the User Service would keep trying to contact it, which could cause more problems. With a circuit breaker, the User Service stops trying to connect to the Order Service, allowing it to keep working smoothly while the Order Service is fixed. This way, your system stays healthy and responsive.

7. Monitoring and Logging
Ever wondered how big companies know immediately when something goes wrong with their services? The secret lies in robust monitoring and logging. Let’s break this down into plain English.
Think of monitoring and logging as your application’s health tracking system – like a Fitbit for your software. Without it, you’re essentially flying blind. You wouldn’t know if your services are struggling until they completely fail, and by then, it’s usually too late.
So what tools can help you keep an eye on things? Here are some popular options that make monitoring a breeze:
Spring Boot Actuator: Provides production-ready features such as metrics, health checks, and application information.
Prometheus: A monitoring system and time series database designed for reliability and scalability.
Grafana: A visualization tool that integrates with Prometheus to create dashboards for monitoring metrics.
ELK Stack: Elasticsearch, Logstash, and Kibana for centralized logging and log analysis.
8. Database per Service
Let’s talk about one of the most debated topics in the microservices world – database architecture. If you’re building microservices, you’ve probably wondered whether each service should have its own database. Let me break this down in a way that makes sense.
Think of microservices like apartments in a building. Just as each apartment has its own kitchen, bathroom, and living space (instead of sharing everything with other tenants), each microservice ideally should have its own database. Why? Because it gives each service the freedom to operate independently without stepping on anyone else’s toes.
So what kind of database should you choose? Well, it depends on what you’re storing, but you’ve got two main options:
Relational Databases: Such as MySQL or PostgreSQL for structured data.
NoSQL Databases: Such as MongoDB or Cassandra for unstructured or semi-structured data.
Here’s the cool part: because each service has its own database, you can mix and match. Maybe your user service needs a traditional PostgreSQL database for reliable user data storage, while your logging service might work better with MongoDB for its flexibility in handling various log formats.
Remember though – with great power comes great responsibility. Having separate databases means you’ll need to think about how to handle situations where you need data from multiple services. But that’s a topic for another day (hint: look up saga patterns and event-driven architecture).
9. Security
When we build microservices, ensuring their security is like locking the doors and windows of your house—it’s essential to protect your data and keep everything running smoothly. Here’s a simple breakdown of how it works:
Why is Security Important?
Microservices often handle sensitive data, like passwords or personal information. If that data falls into the wrong hands, it could lead to serious problems. Security measures ensure that only authorized people or systems can access the data and services, keeping them safe from hackers and other threats.
Spring Security: Your Security Guard
Spring Security is a toolkit for Java developers that helps secure applications. Think of it as a customizable security guard for your app. It handles:
- Authentication: Making sure that users are who they say they are.
- Authorization: Checking if users have permission to access specific resources.
- Extra Protection: Guarding against common security risks, like someone trying to steal your login details.
OAuth2 and JWT: Your Digital Keys
Imagine you’re entering a high-security building. OAuth2 and JWT are like your keycards:
- OAuth2: This system allows you to give limited access to your app’s features to others, without sharing your password. For example, letting a payment app access your bank account to complete a transaction.
- JWT (JSON Web Tokens): These are secure, tamper-proof “passes” that let services communicate safely. When a user logs in, the system creates a JWT. Every time the user makes a request, they show this token to prove they’re authorized.
10. Containerization and Orchestration
Building and managing applications can get tricky, especially when they grow big. That’s where tools like Docker and Kubernetes come in, making everything smoother and more efficient.
Containerization
Think of a container as a portable box that holds your app and everything it needs to run—like its code, libraries, and settings. This means the app will work exactly the same whether you’re running it on your laptop or on a server in the cloud. Docker is a popular tool that creates these containers. It’s like a factory where you package your app in a neat little box.
Why Use Containers?
- Consistency: Developers can run the app the same way on their computer as it runs in production.
- Easy Deployment: You can quickly move the container from one place to another without worrying about setup issues.
- Scalability: Need more instances of your app? Just make more copies of the container!
Orchestration
Managing one or two containers is easy. But what if you have hundreds? Orchestration tools like Kubernetes step in to help manage all those containers. Kubernetes is a platform that automates how containers are deployed, scaled, and managed. It’s like having a smart manager who knows when to hire more help (scale up), let some go (scale down), or handle issues (rollbacks).
The Benefits of Using Docker and Kubernetes Together
- Smooth Deployments: Move your app from development to production without headaches.
- Efficient Scaling: Handle more traffic by spinning up more containers automatically.
- High Availability: If something fails, Kubernetes ensures your app keeps running smoothly.
By using Docker and Kubernetes, you’re giving your applications a rock-solid foundation to grow, adapt, and perform at their best.
11. Event-Driven Architecture
Imagine a system where services talk to each other by passing notes instead of having direct conversations. That’s what Event-Driven Architecture (EDA) is all about! It’s a smart way to design systems where microservices communicate by sharing events. Here’s a simple breakdown
In EDA, services don’t rely on each other directly. Instead, they use events to send and receive updates. An event is just a message that says, “Hey, something important happened!”
For example:
- A user places an order. This triggers an OrderPlaced event.
- The OrderPlaced event is picked up by other services like inventory management (to update stock) or notifications (to send a confirmation email).
An event-driven system works like this:
- Event Producers: These are the services that generate events. For instance, an e-commerce service might produce an OrderPlaced event when an order is submitted.
- Event Consumers: These are the services that listen for events and act on them. For example, an inventory service might reduce stock when it receives the OrderPlaced event.
- Event Bus or Message Broker: This is the middleman that delivers events from producers to consumers.
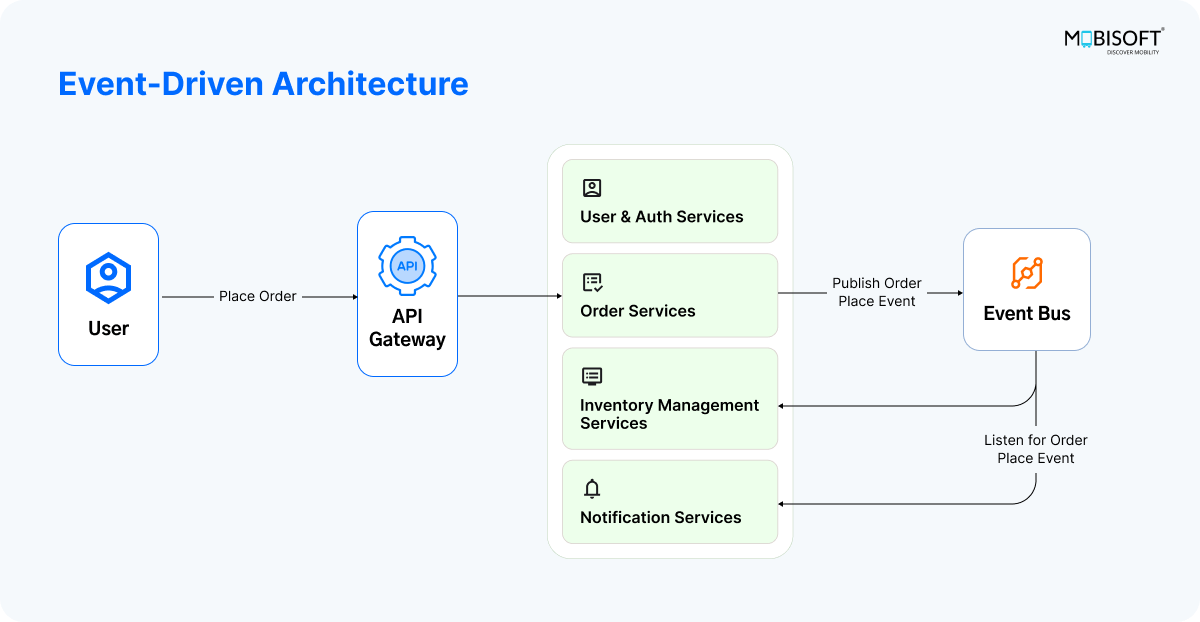
Popular tools include Kafka, RabbitMQ
Apache Kafka: A distributed streaming platform that can be used for building real-time data pipelines and streaming applications. It allows services to publish and subscribe to streams of records.
RabbitMQ: A message broker that facilitates communication between services through message queuing. It supports various messaging patterns, including publish/subscribe and request/reply.
EDA is like a modern, efficient team where everyone knows their job and only gets involved when it’s their turn—making the system faster, more flexible, and easier to manage!
Conclusion
Microservices architecture, powered by Spring Boot, is a game-changer for building modern, scalable, and resilient applications. By breaking down complex systems into smaller, independent services, microservices promote agility, scalability, and fault isolation. Spring Boot further simplifies this journey with its developer-friendly features, robust ecosystem, and seamless integration capabilities.
As businesses increasingly shift toward cloud-native architectures, the combination of Spring Boot and microservices offers a powerful solution to meet evolving demands. Whether you’re building new applications or modernizing legacy systems, adopting these technologies can set your organization on the path to innovation and success.

Author's Bio:
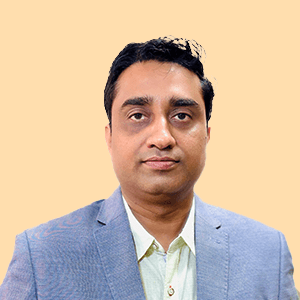
Pratik Kale leads the Web and Cloud technology team at Mobisoft Infotech. With 14 years of experience, he specializes in building complex software systems, particularly focusing on backend development and cloud computing. He is an expert in programming languages like Java and Node.js, and frameworks like Spring Boot. Pratik creates technology solutions that not only solve current business problems but are also designed to handle future challenges.