Grouped table view is an extension of the normal table view in iPhone. It displayes the data in several sectioned lists. Each section has a header and number of rows associated with it. Examples of grouped table are the ‘contacts’ list, a dictionary,etc. In this tutorial, we will create a grouped table using a dictionary, to create a names list,unlike the plain table in which we had used an array. So lets get started!
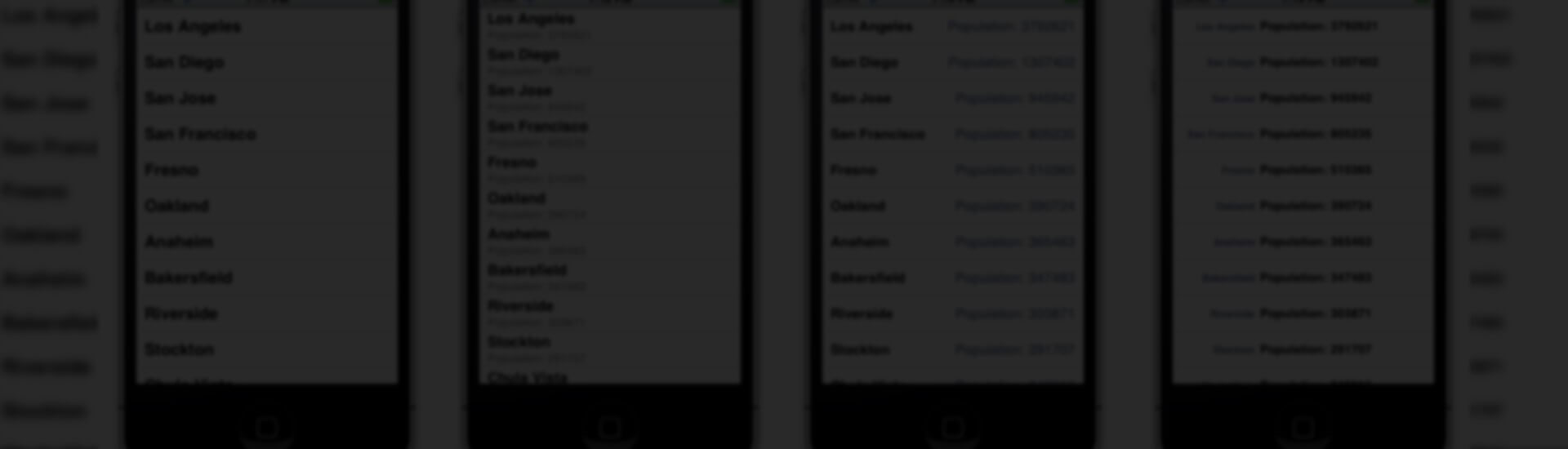
Step 1: Create a view based application and name it ‘GroupedTable’.
Step 2: Put the following code in ‘GroupedTableViewController.h’
[objc highlight="4,5,8,9"] #import <UIKit/UIKit.h> @interface GroupedTableViewController : UIViewController { NSDictionary *tableContents; NSArray *sortedKeys; } @property (nonatomic,retain) NSDictionary *tableContents; @property (nonatomic,retain) NSArray *sortedKeys; @end [/objc]
Here, we have declared a dictionary to hold the section headers and their respective row contents. And an array to hold the sorted keys of the dictionary.
Step 3: Now, open ‘GroupedTableViewController.m’ file and put the following code in it.
[objc highlight="4,5,9,10,11,12,13,14,15,16,17,18,19,20,21,22,23,24,25,29,30,34,36,37,38,39,40,41,42,43,44,45,46,47,48,49,50,51,52,53,54,55,56,57,58,59,60,61,62,63,64,65,66,67,68,69,70,71,72,73,74,75,76,77,78,79,80,81,82,83,84,85,86,87,88,89,90,91,92,93,94,95,96"] #import "GroupedTableViewController.h" @implementation GroupedTableViewController @synthesize tableContents; @synthesize sortedKeys; // Implement viewDidLoad to do additional setup after loading the view, typically from a nib. - (void)viewDidLoad { NSArray *arrTemp1 = [[NSArray alloc] initWithObjects:@"Andrew",@"Aubrey",@"Alice",nil]; NSArray *arrTemp2 = [[NSArray alloc] initWithObjects:@"Bob",@"Bill",@"Bianca",nil]; NSArray *arrTemp3 = [[NSArray alloc] initWithObjects:@"Candice",@"Clint",@"Chris",nil]; NSDictionary *temp =[[NSDictionary alloc] initWithObjectsAndKeys:arrTemp1,@"A",arrTemp2, @"B",arrTemp3,@"C",nil]; self.tableContents =temp; [temp release]; self.sortedKeys =[[self.tableContents allKeys] sortedArrayUsingSelector:@selector(compare:)]; [arrTemp1 release]; [arrTemp2 release]; [arrTemp3 release]; [super viewDidLoad]; } - (void)dealloc { [tableContents release]; [sortedKeys release]; [super dealloc]; } #pragma mark Table Methods - (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView{ return [self.sortedKeys count]; } - (NSString *)tableView:(UITableView *)tableView titleForHeaderInSection:(NSInteger)section { return [self.sortedKeys objectAtIndex:section]; } - (NSInteger)tableView:(UITableView *)table numberOfRowsInSection:(NSInteger)section { NSArray *listData =[self.tableContents objectForKey: [self.sortedKeys objectAtIndex:section]]; return [listData count]; } - (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath { static NSString *SimpleTableIdentifier = @"SimpleTableIdentifier"; NSArray *listData =[self.tableContents objectForKey: [self.sortedKeys objectAtIndex:[indexPath section]]]; UITableViewCell * cell = [tableView dequeueReusableCellWithIdentifier: SimpleTableIdentifier]; if(cell == nil) { cell = [[[UITableViewCell alloc] initWithStyle:UITableViewCellStyleDefault reuseIdentifier:SimpleTableIdentifier] autorelease]; /*cell = [[[UITableViewCell alloc] initWithStyle:UITableViewCellStyleSubtitle reuseIdentifier:SimpleTableIdentifier] autorelease]; */ } NSUInteger row = [indexPath row]; cell.textLabel.text = [listData objectAtIndex:row]; return cell; } - (void)tableView:(UITableView *)tableView didSelectRowAtIndexPath:(NSIndexPath *)indexPath { NSArray *listData =[self.tableContents objectForKey: [self.sortedKeys objectAtIndex:[indexPath section]]]; NSUInteger row = [indexPath row]; NSString *rowValue = [listData objectAtIndex:row]; NSString *message = [[NSString alloc] initWithFormat:rowValue]; UIAlertView *alert = [[UIAlertView alloc] initWithTitle:@"You selected" message:message delegate:nil cancelButtonTitle:@"OK" otherButtonTitles:nil]; [alert show]; [alert release]; [message release]; [tableView deselectRowAtIndexPath:indexPath animated:YES]; } @end [/objc]
viewdidLoad: Here, we populate the dictionary using three arrays of names as objects and alphabets as keys. Then we sort the array ‘allKeys’ of the dictionary and put it in sortedKeys.
Table Methods
numberOfSectionsInTableView : Returns the count of keys in the dictionary.
titleForHeaderInSection : Returns the value of key for that particular section.
cellForRowAtIndexPath : Here, listdata is an array which is assigned the value for the key corresponding to the respective section. For every section, the contents of listdata are displayed.
didSelectRowAtIndexPath : In this method, we display an alert view when a row is selected.
Step 4 : Open the ‘GroupedTableViewController.xib’. Drag and drop a table view on it. Select the table view and open its Attributes Inspector (command+1). Change the table style from ‘Plain’ to ‘Grouped’.
Step 5 : Open the Connections Inspector (command+2) for the table and link its datasource and delegate to the file’s owner.
Step 6 : You can download the source code here.Save,build and run the project. The output will be as follows: