What is CARD.IO ?
The card.io provides friendly API’s and methods ready to use. With card.io, you can scan the credit card details like card no, exp. date, name of cardholder in your iOS / Android app in a fast and easy way.
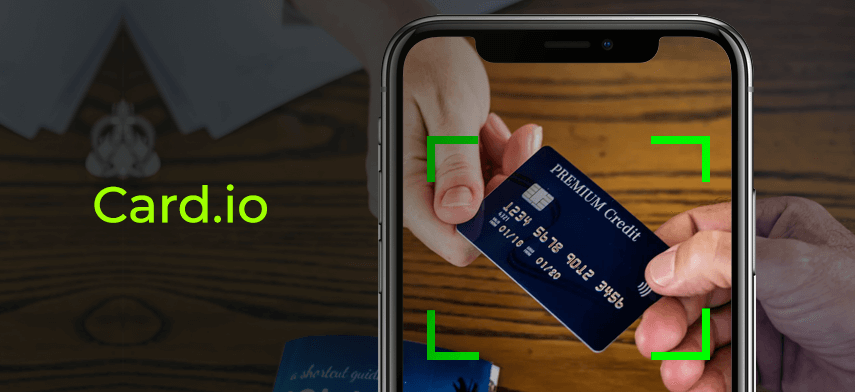
Getting Started
1. Download the starter project ‘CardIO_Starter_Project’ from here and open it in the Xcode.
2. Next step = Do you use cocoa pods ? Yes, Jump to step #5 : No, Jump to step #3;
3. Download the latest version of card.io SDK from here.
4. Add the ‘CardIO’ directory into the project and skip the next step.
5. Simply add pod ‘CardIO’ into the podfile and update.
6. Go to the TARGET -> BUILD SETTINGS and set OTHER LINKER FLAG to “-lc++” and “-ObjC”
7. Add following frameworks as Optional libraries
- Accelerate
- AVFoundation
- AudioToolbox
- CoreMedia
- MobileCoreServices
8. Finally, Confirm that following two settings ( TARGET->BUILD SETTINGS ) are enabled, if not enable them
- Enable Modules (C and Objective-C)
- Link Frameworks Automatically
Using Objective-C into Swift Project
The Card.io framework is written in Objective-C, and our project is written in Swift. These two don’t work together without a proper configuration. Any Objective-C library, project or class can be used in a Swift project by setting up a bridging header.
You can create the bridging file by using following steps,
1. Add a new Header file into project, using File – > New -> File -> iOS -> Source -> Header File.
2. Name the class <your-project-name>-Bridging-Header.h and press continue to save the file.
3. Go to Build Settings -> Objective-C Bridging Header and set as ‘<your-project-name>-Bridging-Header.h’.
4. Add “#import “CardIO.h” in to your bridging header file.
Using Card.io
There are two ways to use the card.io in to the project, Choose as per your requirement
Ready Made ( As a View Controller )
As the title suggest, it is ready to use controller and it is very quick and easy. What you have to do is, Just create a instance of a class ‘CardIOPaymentViewController’ and present it modally, And it will handle rest all.
Do It Yourself ( As a View )
If you are the DIY kind of developer and want to more control over the transitions and presentation of the scanner, then you should use this approach. This will only provide the magic for the scanning the card, rest handle yourself. You have to create a instance of ‘CardIOView’ which will handle the scanning.
In this tutorial, I will cover the only the Ready Made approach, because most of us not really required the modifications in the scanner but we will cover DIY approach in the next part of this tutorial.
Integrating As a View Controller
- Open ‘ReadyMadeViewController.swift’ class file from our ‘CardioDemoStarter’ project. And add CardIOPaymentViewControllerDelegate to the class.
class ReadyMadeViewController: UIViewController,
CardIOPaymentViewControllerDelegate {
}
- The first time that you create either a CardIOPaymentViewController or a CardIOView, the card.io SDK must load resources, which can result in a noticeable delay. To avoid this delay you may optionally call CardIOUtilities.preload() in advance, so that this resource loading occurs in advance on a background thread.
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
CardIOUtilities.preload()
}
- Now create a actual scanner and start the scanning add the following code in the “scanButtonClicked” action.
/// Create CardIOPaymentViewController object with paymentDelegate to self.
let cardIOVC = CardIOPaymentViewController(paymentDelegate: self)
- Also there are few optional configurations in the scanner.
/// Set to true if you need to collect the cardholder name. Defaults to false.
cardIOVC.collectCardholderName = true
/// Hide the PayPal or card.io logo in the scan view. Defaults to false.
cardIOVC.hideCardIOLogo = true
/// Set the guide color, Defaults to nil; if nil, will use card.io green.
cardIOVC.guideColor = UIColor.redColor()
/// Set to false if you don't need to collect the CVV from the user. Defaults to true.
cardIOVC.collectCVV = true
- Finally present the scanner modally.
/// Present Card Scanner View modally.
presentViewController(cardIOVC, animated: true, completion: nil)
- Now, Implement the delegate method to get card info or cancellation.
func userDidCancelPaymentViewController(paymentViewController: CardIOPaymentViewController!) {
labelResults.text = "User has canceled the scanner."
paymentViewController.dismissViewControllerAnimated(true, completion: nil)
}
func userDidProvideCreditCardInfo(cardInfo: CardIOCreditCardInfo?,
inPaymentViewController paymentViewController: CardIOPaymentViewController!) {
if let info = cardInfo {
let str = NSString(format: "Received card info.\n Cardholders Name: %@ \n Number: %@\n expiry: %02lu/%lu\n cvv: %@.", info.cardholderName, info.
redactedCardNumber, info.expiryMonth, info.expiryYear, info.cvv)
l abelResults.text = str as String
}
paymentViewController.dismissViewControllerAnimated(true, completion: nil)
}
Build and Run
You may download the complete project ‘CardIO_Complete_Project’ from here.
Happy Coding!
Written by – Shrimant Nikate.
iOS Developer – Mobisoft Infotech.