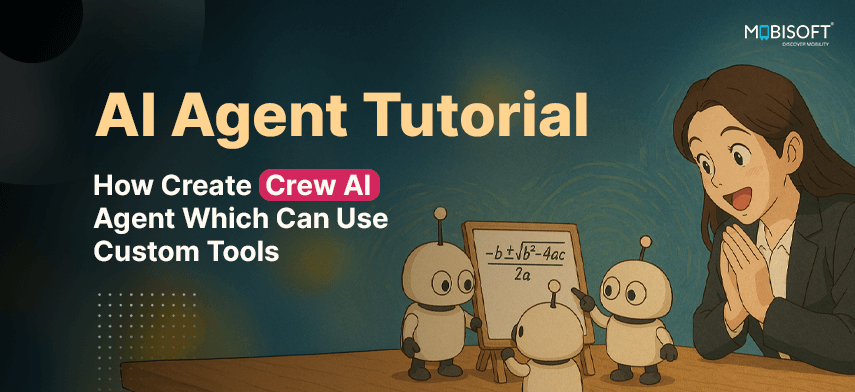
In the last tutorial we saw how we can create an AI agent with Crew AI in pure python code. It’s advised for the reader to check that tutorial out to understand how Crew AI agents work at the code level.
As your AI agents become more ambitious, you need proper structure to develop custom AI agents. The CrewAI framework offers a CLI utility that allows you to generate the scaffolding to jumpstart the creation of your AI agent. In this tutorial, we will create a simple Math Agent to demonstrate how to create AI agents using CrewAI CLI. Also, while developing custom AI agents, it’s a common requirement that you need to perform functions that the traditional LLM can’t perform. To solve this, LLMs have introduced a concept of “Tool Calling” or “Function Calling”. Function Calling provides LLMs the power to perform tasks beyond just generating textual output. In this blog, we will also create a custom tool that the LLM can use to solve mathematical expressions. For businesses looking to streamline their AI integration, you may want to explore AI strategy consulting services, which can help align your AI efforts with long-term goals.
Note: All the code for this tutorial is present at this Github repo.
Installation
CrewAI requires Python >=3.10 and <3.13. Here’s how to check your version:
python3 --version
Code language: Python (python)
CrewAI
uses UV to manage dependencies. So we need to install UV. On Mac OS or Linux, you can use:
curl -LsSf https://astral.sh/uv/install.sh | sh
Code language: Python (python)
Once the uv command is installed, you can use the following command to install the crewai
CLI.
uv tool install crewai
Code language: Python (python)
Now, we can create our project
crewai create crew math-agent
Code language: Python (python)
Select ‘OpenAI’ as the provider and choose ‘gpt-4o’ as the model. Since we are going to use function calling in this tutorial ,it’s important to choose a model that has function calling capabilities.
This should create a directory structure like the following
math_agent
├── README.md
├── knowledge
│ └── user_preference.txt
├── pyproject.toml
├── src
│ └── math_agent
│ ├── __init__.py
│ ├── config
│ │ ├── agents.yaml
│ │ └── tasks.yaml
│ ├── crew.py
│ ├── main.py
│ └── tools
│ ├── __init__.py
│ └── custom_tool.py
└── tests
7 directories, 10 files
Code language: Python (python)
pyproject.toml
A standardized configuration file that holds project metadata, dependencies, and build configuration. It’s used by modern Python packaging tools (like uv) to manage your project environment.
Directory src/math_agent/
config/
Contains YAML configuration files that define how your AI agents and their tasks behave:
- agents.yaml
Specifies the configuration for each agent (roles, goals, backstories, LLM settings, etc.). This file lets you customize the personality and behavior of your agents. - tasks.yaml
Defines the tasks that the agents will perform. It includes descriptions, expected outputs, and assigns tasks to specific agents. This helps set the workflow for your project.
crew.py
Contains the core logic that assembles the crew. Here you define how agents and tasks are orchestrated, including the process (e.g., sequential or parallel) and any custom logic or tool integration.
main.py
Acts as the entry point to run your project. It typically sets up necessary inputs (like user-defined variables), initializes the crew defined in crew.py, and kicks off the execution process.
Tools Directory in Building AI Agents (src/math_agent/tools/)
__init__.py
Marks the tools folder as a Python package, enabling you to import your custom tools into your crew logic.
custom_tool.py
A placeholder file where you can implement custom tools. These tools extend the functionality of your agents by providing specialized operations or integrations not available in the core framework.
For instance, if you need a conversational AI to handle SQL queries or interact with databases, you can integrate a SQL AI agent. You can dive deeper into how to implement SQL AI agent in this Conversational Analytics Tutorial: How to Implement SQL AI Agent that Speaks Database.
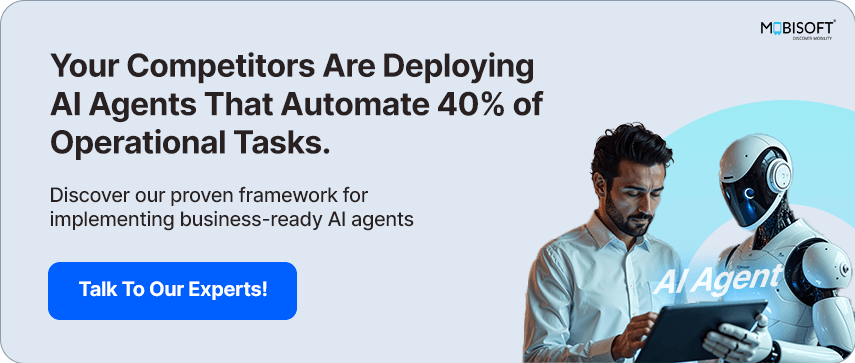
Let’s Build Custom AI Agent!
Open the math_agent/src/math_agent/config/agents.yaml
and replace its code with the following:
math_expert:
role: >
Math Expert
goal: >
Solve math problems
backstory: >
You're a math expert with a knack for solving math problems.
When the user asks you to solve a math problem, you should use the math_tool to solve the problem.
Code language: Python (python)
Here, as you can see, it’s important to give precise instructions so that the LLM will understand those clearly.
Next Open math_agent/src/math_agent/config/tasks.yaml
and replace its code with the following:
math_task:
description: >
Solve the math problem {problem}
Make sure to use the math_tool to solve the problem
Before passing the problem to the math_tool, make sure to convert the problem in normal english to a pure mathematical expression.
For example:
"What is the sum of 2 and 2?" should be converted to "2 + 2"
"What is the product of 2 and 2?" should be converted to "2 * 2"
"What is the difference between 4 and 2?" should be converted to "4 - 2"
"What is the quotient of 4 and 2?" should be converted to "4 / 2"
expected_output: >
The solution to the problem {problem}
agent: math_expert
Code language: Python (python)
It’s very important to be precise in your task description. Also make sure to give examples wherever possible.
Next, open math_agent/src/math_agent/tools/custom_tool.py
and replace its code with the following:
from crewai.tools import tool
@tool("math_tool")
def math_tool(problem: str) -> str:
"""This tool is used to solve math problems."""
return eval(problem)
Code language: Python (python)
Here the main thing to note is the `@tool("math_tool")
` decorator which registers the following function as a tool with the identifier “math_tool
“. This means that within the CrewAI ecosystem, this function can be dynamically discovered and invoked by agents as a utility to solve math problems.
Note: Here for the sake of keeping the code simple, we are directly using “eval” on LLM generated expressions from the user input. This is not safe in production. Make sure to add proper safeguards for production situations by properly considering the threat vectors.
For more information on how AI is being applied to solve complex business problems, check out Artificial Intelligence consulting Services.
Next, let’s put this all together as a crew. Open the math_agent/src/math_agent/crew.py
file and add the following code:
from crewai import Agent, Crew, Process, Task
from crewai.project import CrewBase, agent, crew, task
from math_agent.tools.custom_tool import math_tool
@CrewBase
class MathAgent():
"""MathAgent crew"""
agents_config = 'config/agents.yaml'
tasks_config = 'config/tasks.yaml'
@agent
def math_expert(self) -> Agent:
config = self.agents_config['math_expert']
config['tools'] = [math_tool]
return Agent(
config=config,
verbose=True
)
@task
def math_task(self) -> Task:
config = self.tasks_config['math_task']
config['tools'] = [math_tool]
return Task(
config=config,
)
@crew
def crew(self) -> Crew:
"""Creates the MathAgent crew"""
return Crew(
agents=self.agents,
tasks=self.tasks,
process=Process.sequential,
verbose=True,
)
Code language: Python (python)
Let me explain the code block by block.
agents_config = 'config/agents.yaml'
tasks_config = 'config/tasks.yaml'
Code language: Python (python)
Here, we are creating 2 instance variables to point to the YAML config files shown earlier.
@agent
def math_expert(self) -> Agent:
config = self.agents_config['math_expert']
config['tools'] = [math_tool]
return Agent(
config=config,
verbose=True
)
Code language: Python (python)
Here we load the config for the `math_expert` and give the math_tool access to it.
@task
def math_task(self) -> Task:
config = self.tasks_config['math_task']
config['tools'] = [math_tool]
return Task(
config=config,
)
Code language: Python (python)
Then we load the config for the math_task
and give the math_tool
access to it.
@crew
def crew(self) -> Crew:
"""Creates the MathAgent crew"""
return Crew(
agents=self.agents,
tasks=self.tasks,
process=Process.sequential,
verbose=True,
)
Code language: Python (python)
Here we are assembling our crew. Now let’s create the main file to run the agent: math_agent/src/math_agent/main.py
#!/usr/bin/env python
import warnings
from math_agent.crew import MathAgent
warnings.filterwarnings("ignore", category=SyntaxWarning, module="pysbd")
def run():
"""
Run the crew.
"""
inputs = {
'problem': 'What is the sum of 3 and 5?',
}
try:
MathAgent().crew().kickoff(inputs=inputs)
except Exception as e:
raise Exception(f"An error occurred while running the crew: {e}")
Code language: Python (python)
Here the run()
method is being used to create an instance of our MathCrew
and to start it. We pass our math question in the input
dict as problem
.
To run the code type in terminal:
crewai run
Code language: Python (python)
This should run your AI agent. It will print some debugging information. Somewhere there you should see something like this:
# Agent: Math Expert
## Final Answer:
The solution to the problem What is the sum of 3 and 5? is 8.
Code language: Python (python)
Conclusion
In this tutorial, we explored how to leverage the Crew AI framework to building AI agents capable of solving mathematical problems. By utilizing the Crew AI CLI, we created a well-organized project structure that not only simplifies the development process but also enhances the maintainability of our AI agents as they grow in complexity.
We also introduced the concept of “Tool Calling,” which empowers our custom AI agents to perform tasks that go beyond mere text generation. By implementing a custom tool for mathematical operations, we demonstrated how to extend the capabilities of our AI agents, making them more functional and versatile.
As we continue exploring the potential of AI, whether for mathematical problem-solving or more advanced applications, remember that AI is constantly evolving
If you’re ready to take the next step in implementing AI solutions for your business or project, Mobisoft Infotech offers AI strategy consulting services to help you craft a tailored AI strategy that aligns with your specific needs and goals. Our team is dedicated to helping you unlock the true potential of AI and drive impactful results for your business.
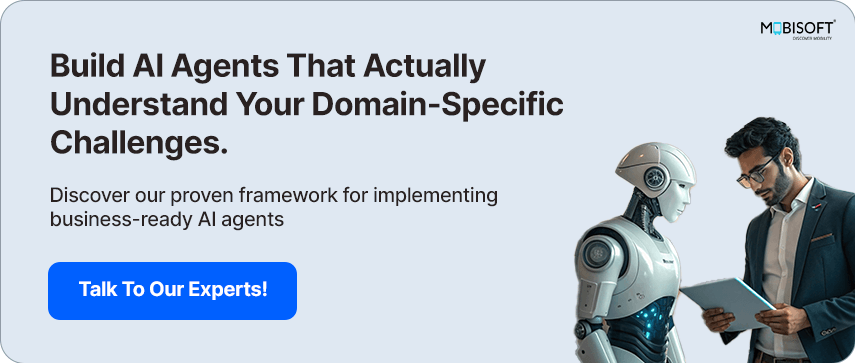
Author's Bio:
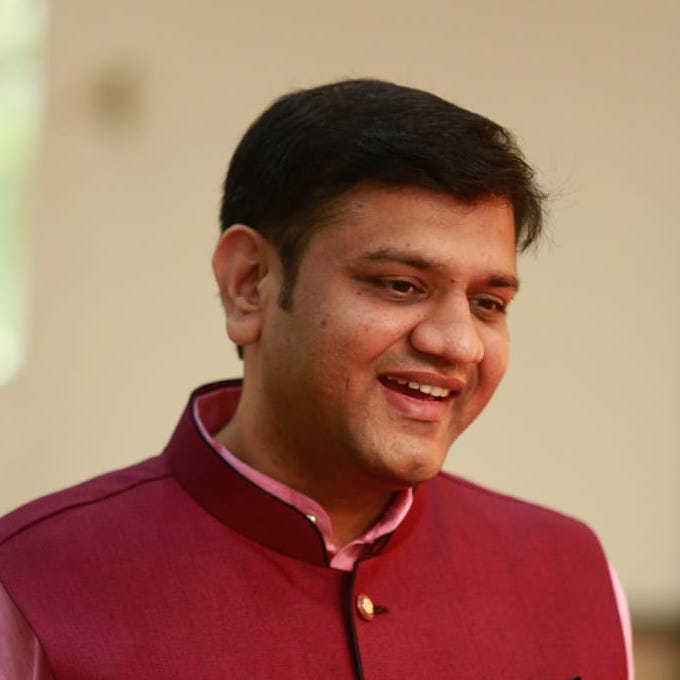
Pritam Barhate, with an experience of 14+ years in technology, heads Technology Innovation at Mobisoft Infotech. He has a rich experience in design and development. He has been a consultant for a variety of industries and startups. At Mobisoft Infotech, he primarily focuses on technology resources and develops the most advanced solutions.