With HTML5 you can access the geolocation of the user.
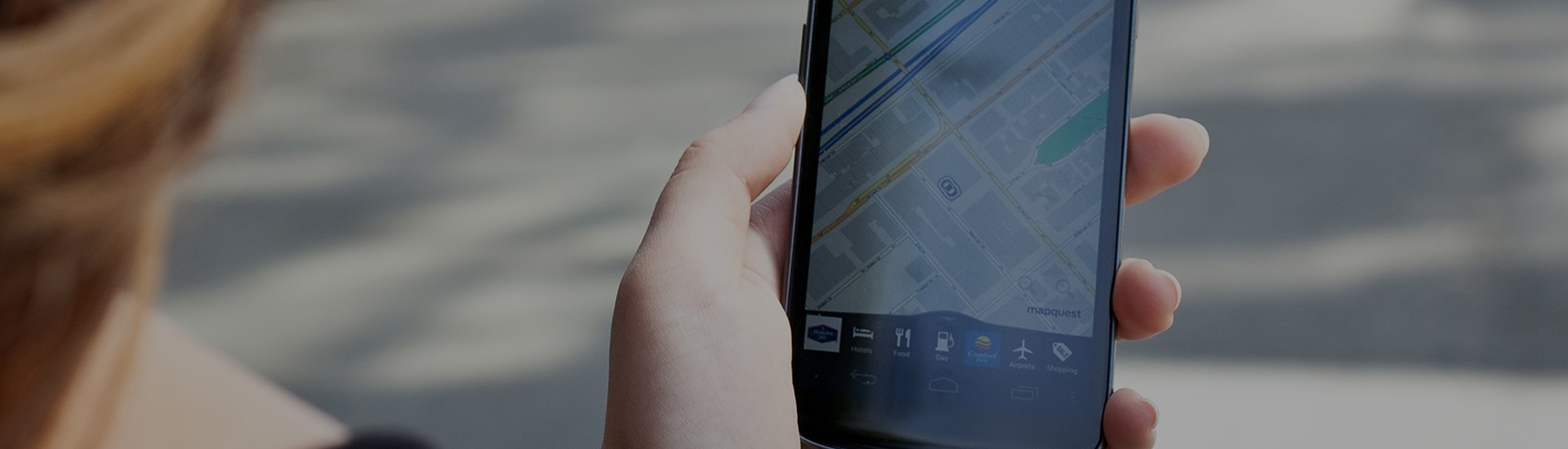
Browser wise Geolocation Support
- Internet Explorer : 9.0 and above
- Chrome : 5.0 and above
- Firefox : 3.5 and above
- Safari : 5.0 and above
- Opera : 10.6 and above
- iPhone : 3.0 and above
- Android : 2.0 and above
- Blackberry : 6.0 and above
- Windows Phone7 : Mango and above[*]
Detecting Geolocation Support
You can use the Modernizr JavaScript library to check if the user’s browser supports geolocation.
[js] if(Modernizr.geolocation) { // HTML5 geolocation supported } else { // HTML5 geolocation not supported } [/js]
Using Geolocation with HTML 5
Here is a small sample you can use to get started with geolocation in HTML5.
If you are testing this locally on your machine, then you will need to use a local web server to run this sample.
[jscript] <!DOCTYPE html> <html> <head> <title>HTML 5 Geolocation Sample Page</title> <script src="modernizr-2.5.2.js"></script> <script src="jquery-1.6.1.min.js"></script> <script type="text/javascript"> var jQ = jQuery.noConflict(); function useGeolocation(position) { var latitude = position.coords.latitude; var longitude = position.coords.longitude; jQ("#latitude").html(latitude); jQ("#longitude").html(longitude); } function onGeolocationError(error) { alert("Geolocation error - code: " + error.code + " message : " + error.message); } jQ(document).ready(function(){ if(Modernizr.geolocation) { jQ("#geolocation").html("is supported."); navigator.geolocation.getCurrentPosition( useGeolocation, onGeolocationError); } else { jQ("#geolocation").html("is not supported."); } }); </script> </head> <body> <b>Geolocation</b> : <span id="geolocation"></span> <br /> <b>Latitude</b> : <span id="latitude"></span> <br /> <b>Longitude</b> : <span id="longitude"></span> <br /> </body> </html> [/jscript]
Screen Shots
Success: User allowed the geolocation access
Error: User denied the geolocation access
Example Source Code
You can download the HTML5 Gelolocation Example source code from here.