Geofences are virtual regions considered around actual geo point with some radius. This geopoint can be considered as any real place like store, restaurant, schools, home place etc. and circular area of that place becomes radius of geo fence. Location services based mobile apps always have been centre of mobile industry and also need. Geofences / geo regions have added spice to it to make more accurate location based and useful mobile applications.
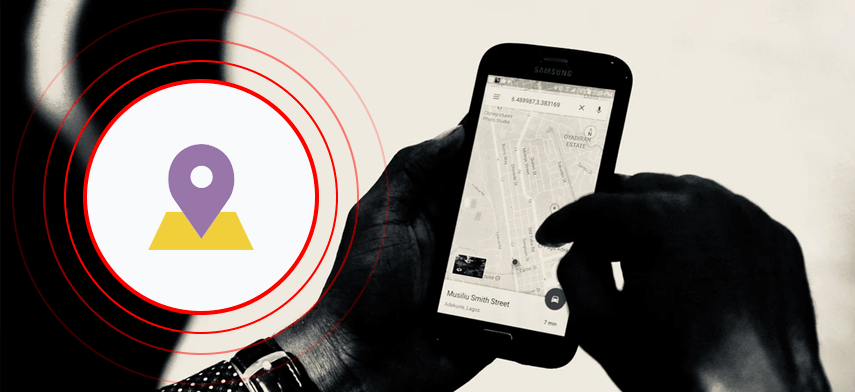
Google has made geofences implementation easy for all android developers.
Pre requisite for geo fences
In order to implement geo fences android device need updated Google Play services installed on it. Before creating or handling geo fences, app should check for Google Play Services installed on device. Without it, geo fences won’t work.
Permission needed to set in manifest file of Android app is
[xml][/xml]
Google Play service is available or not can be checked using following code snippet
private boolean servicesConnected() { // Check that Google Play services is available int resultCode = GooglePlayServicesUtil. isGooglePlayServicesAvailable(this); // If Google Play services is available if (ConnectionResult.SUCCESS == resultCode) { // In debug mode, log the status Log.d("Geofence Detection","Google Play services is available."); // Continue return true; // Google Play services was not available for some reason } else { // Get the error code int errorCode = connectionResult.getErrorCode(); // Get the error dialog from Google Play services Dialog errorDialog = GooglePlayServicesUtil.getErrorDialog(errorCode, this, CONNECTION_FAILURE_RESOLUTION_REQUEST); // If Google Play services can provide an error dialog if (errorDialog != null) { // Create a new DialogFragment for the error dialog ErrorDialogFragment errorFragment = new ErrorDialogFragment(); // Set the dialog in the DialogFragment errorFragment.setDialog(errorDialog); // Show the error dialog in the DialogFragment errorFragment.show(getSupportFragmentManager(), "Geofence Detection"); } }
Creating geo fences
After requesting or checking Google Play Services, geo fences can be created. Geo fence object has following parameter which values can be set while creating geo fence.
- — Latitude of geo point around which geo fence has to be created
- — Longitude of geo point around which geo fence has to be created
- — Radius of geofence to be created around choosen geo fence
- — Geo fence expiration time – (App monitors geo fences created for the expiration time set. If it is not set, app will monitor geo fences throughout app life cycle)
- — Event to be monitored for geo fence. It could be either entry or exit event of geo fence.
- — GeofenceStorage needs to be initialized as
SimpleGeofenceStore mGeofenceStorage = new SimpleGeofenceStore(this);
- Geo fence can be created using following code snippet
SimpleGeofence mUIGeofence1 = new SimpleGeofence( "1", Double.valueOf(mLatitude1.getText().toString()), Double.valueOf(mLongitude1.getText().toString()), Float.valueOf(mRadius1.getText().toString()), GEOFENCE_EXPIRATION_TIME, // This geofence records only entry transitions Geofence.GEOFENCE_TRANSITION_ENTER); mGeofenceStorage.setGeofence("1", mUIGeofence1);
This way multiple geo fences can be added in GeofenceStore for multiple geo places. Every geo fence created is identified with unique id used while setting geo fence in GeofenceStorage as shown above.
Start Monitoring Geo Fence tracking
Once geo fences are created, app can start monitoring those geo fences using getTansitionPendingIntent. Pending intents are intialized in onConnect method of ConnectionsCallback as follows
@Override private void onConnected(Bundle dataBundle) { ... switch (mRequestType) { case ADD : // Get the PendingIntent for the request mTransitionPendingIntent = getTransitionPendingIntent(); // Send a request to add the current geofences mLocationClient.addGeofences( mCurrentGeofences, pendingIntent, this); ... } }
Transition events detection
Once geofences are created and pending intent is registered, location services detects that user has entered or exited a geofence, it sends out intent contained in pending indent which is included in add geo fence. Custom intent service can be written which is extended from IntentService class and events triggered are handled in onHandleIntent method of it.
// Get the type of transition (entry or exit) int transitionType =LocationClient.getGeofenceTransition(intent); // Test that a valid transition was reported if ((transitionType == Geofence.GEOFENCE_TRANSITION_ENTER) || (transitionType == Geofence.GEOFENCE_TRANSITION_EXIT)) { List <Geofence> triggerList = getTriggeringGeofences(intent); String[] triggerIds = new String[geofenceList.size()]; for (int i = 0; i < triggerIds.length; i++) { // Store the Id of each geofence triggerIds[i] = triggerList.get(i).getRequestId(); } /* * At this point, you can store the IDs for further use * display them, or display the details associated with * them. */ }
Once receiver receives intent telling event triggered local notification can be generated and shown to user.
Stop monitoring geo fences
Monitoring of geo fences can be stopped by removing geo fence themselves from GeoFenceStorage. Once geo fences are removed they no longer exist and monitoring automatically stops for respective geo fences. Code to remove geo fence is as follows
public void removeGeofences(PendingIntent requestIntent) { // Record the type of removal request mRequestType = REMOVE_INTENT; if (!servicesConnected()) { return; } // Store the PendingIntent mGeofenceRequestIntent = requestIntent; mLocationClient = new LocationClient(this, this, this); // If a request is not already underway if (!mInProgress) { // Indicate that a request is underway mInProgress = true; // Request a connection from the client to Location Services mLocationClient.connect(); } }
User can remove multiple geo fences using above source code.
Benefits of using geofences
- — Geo fences implementation can be widely used for location services based apps where app is monitoring some regions like some places.
- — Geo fences can be used to show Location based Ads.
- — As Google Play Services solely takes care of location tracking of regions defines, it does battery optimization at great extend. As Google Play Service is already running on device, every app which implements geo fences monitoring don’t need to write own service for location tracking. So battery saving is great.
Source : http://developer.android.com/training/location/geofencing.html