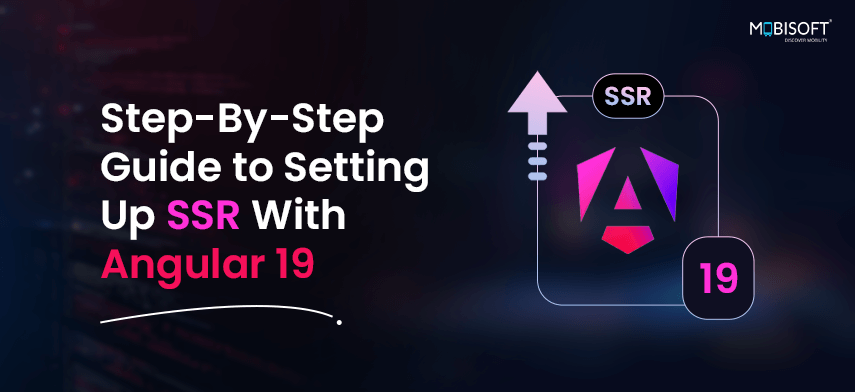
Benefits of Using SSR
Make Web Crawlers Easier (SEO):
Web crawlers are essential for indexing your application’s content and making it searchable on the internet. Your highly interactive Angular application may be too complicated for these web spiders to browse and index.
Without the need for JavaScript, Angular Universal SSR can create a static version of your application that is simple to search, link, and navigate. Because every URL produces a completely rendered page, Angular Universal also provides a site preview.
Enhanced Functionality on Portable and Low-Powered Devices:
The user experience is unsatisfactory on some devices since JavaScript is either not supported or is executed so poorly. In certain situations, you might need a server-rendered version of the application that doesn’t use JavaScript. People who would not otherwise be able to use the app at all could find that this version, despite its limitations, is their sole workable option.
Rapidly Display the First Page:
User engagement may be greatly impacted by how soon the initial page loads. Even with 100ms of change, faster-loading pages perform better. For your app to engage these people before they choose to do something else, it might need to launch more quickly.
You can create app landing pages that mimic the whole app using Angular Universal SSR. Even if JavaScript is disabled, the pages can still be displayed because they are entirely HTML. The pages allow router link navigation through the site, but they do not manage browser events.
A static version of the landing page will be displayed in practice to keep the user’s interest. You’ll load the entire Angular 19 application behind it at the same time. The landing page provides almost instantaneous performance, and after the entire app is loaded, the user can enjoy the complete interactive experience.

Why Use SSR in Angular 19?
- Improved user experience, quicker load times, and a more seamless and engaging experience.
- For improving SEO in Angular applications, Angular Universal is an essential tool. Because of this, Angular apps can be pre-rendered on the server, producing static HTML pages that search engine bots can easily crawl.
- Enhancements to SSR are offered by Angular 19, including support for ESM in server builds, which enables developers to incorporate modules into their server code. It also provides enhancements to the development server’s and server builds’ performance.
Step 1: Node.js Compatibility
Knowing which version of Node.js is compatible with a certain version of Angular is a must because Angular depends so heavily on Node.js. Below is the table indicating version compatibility:
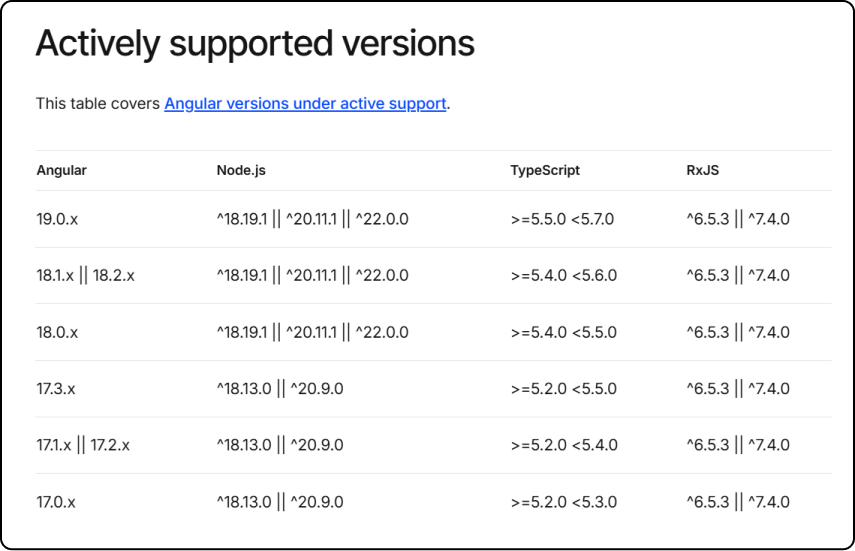
Step 2: Server-Side Rendering (SSR):
Server-Side Rendering (SSR) is a web development approach where the Node server generates the HTML content for a web page, leading to faster initial load times and better SEO performance. Angular Universal SSR uses Express.js to manage backend routing and CommonEngine to render the Angular application on the Express server.When creating a new project, run the following command to set up the server-side rendering configuration for Angular Universal:
$ ng new --ssr
Code language: JavaScript (javascript)
For already created projects, Just run the ng add command:
$ ng add @angular/ssr
Step 3: Default Config Imports
Once you create an Angular SSR project, Angular will create a server.ts which loads the app by using Express.js, and a main.server.ts which functions the same as Angular App Module but for the server.
Ensure all the configuration is correctly set up.
Specifically, the angular.json file should have the server file configured build/options as => “server”: “src/main.server.ts”,
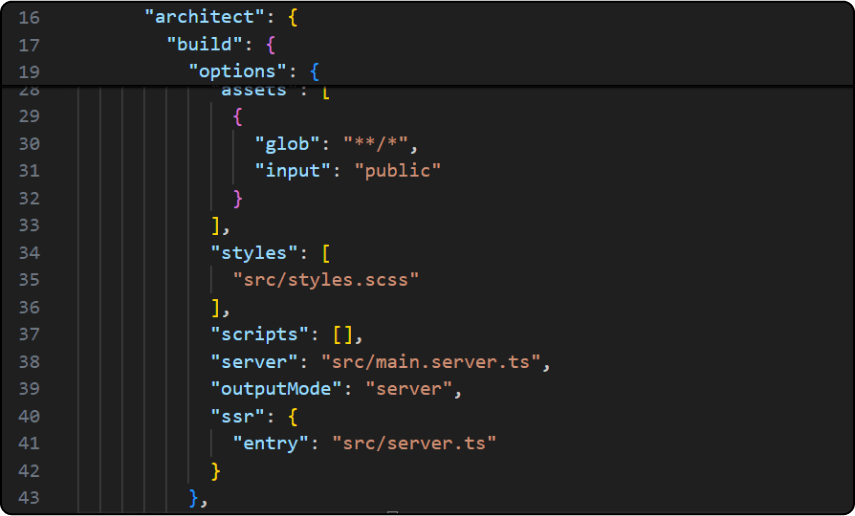
Confirm that the tsconfig.app.json file was added with the below code:
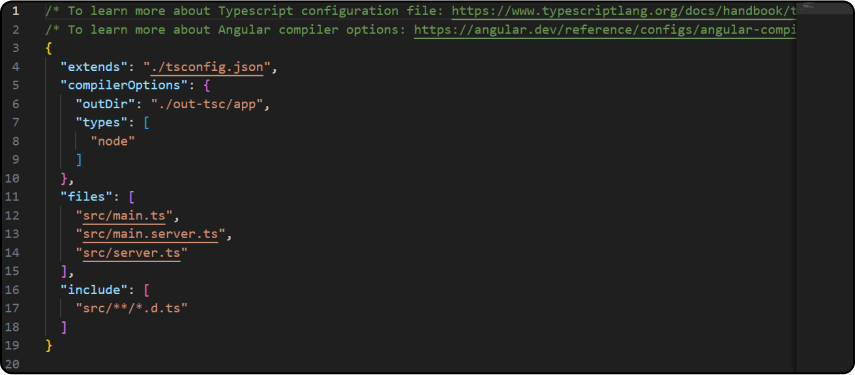
In short:
- app.config.server.ts: Used for server application configuration.
- src/main.server.ts: It serves as the server-side rendering logic’s entry point. We can manage the main server application startup with the aid of this file.
- src/app/app.server.module.ts: Includes the server-side application module for Angular Universal SSR.
- src/server.ts: This file is used by the Express server to render the Angular application on the server-side.
Step 4: Building the App
The default scripts added by SSR in Angular Universal will help you build your application. First, build the app using the build script from the package.json file:
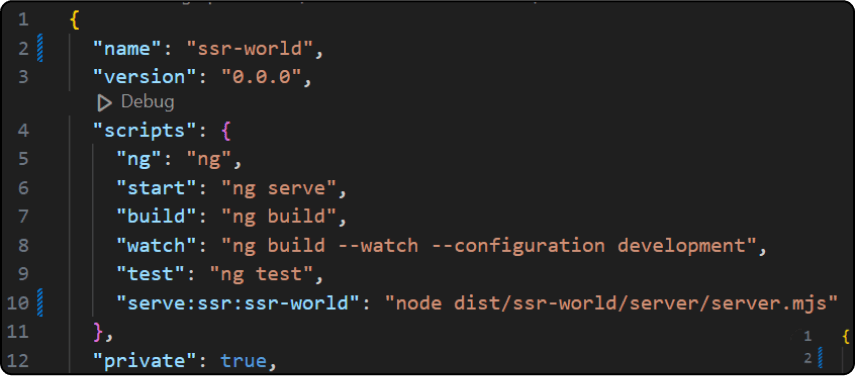
Build the application with the command as ng build
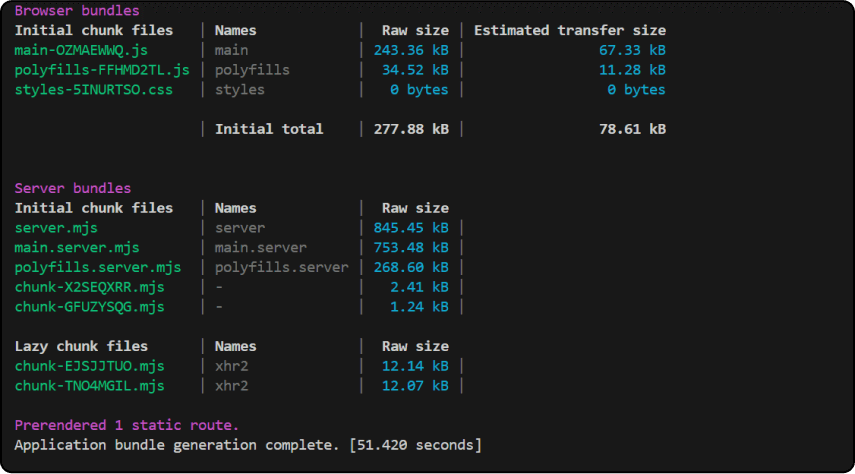
This will generate a dist folder in your directory for both browser and server.
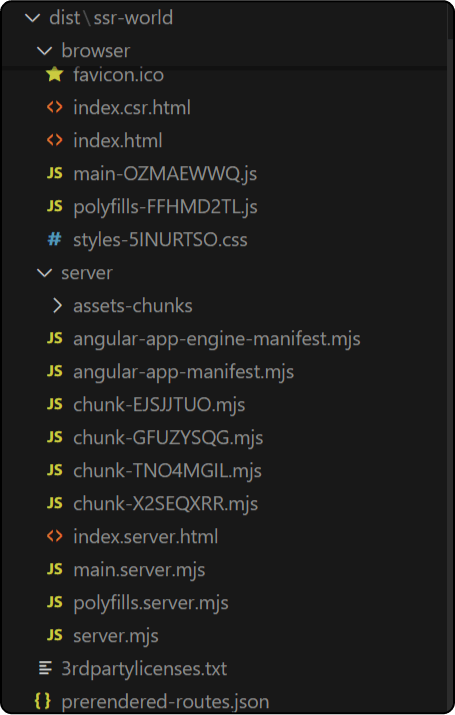
Step 5: Running the App
You can run the server by using the command: node dist/<your-ssr-aap-name>/server/server.mjs

You can see applications running on a given port with the default Angular Framework.
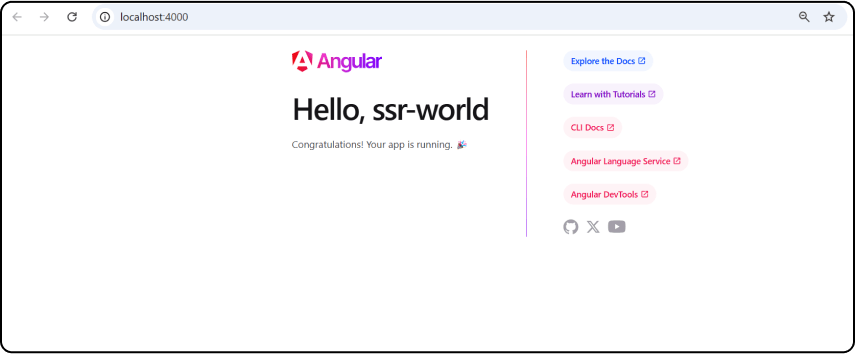
Step 6: Interaction Between Backend and Frontend
To establish the connection between the backend and frontend in Angular Universal SSR, you need to add provideHttpClient(withFetch()) in your app.config.ts file
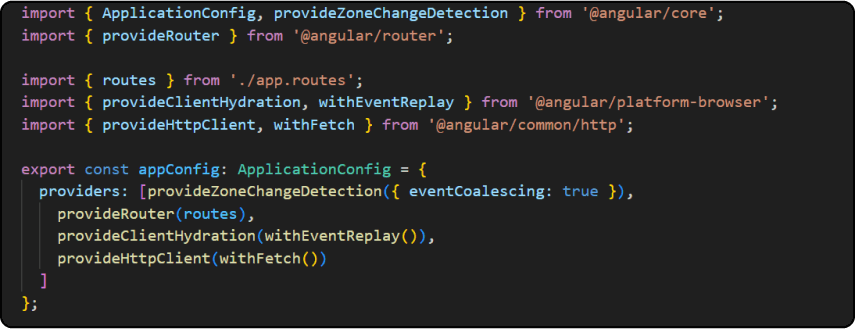
To make it work, create a service with the endpoint in the server.ts file:
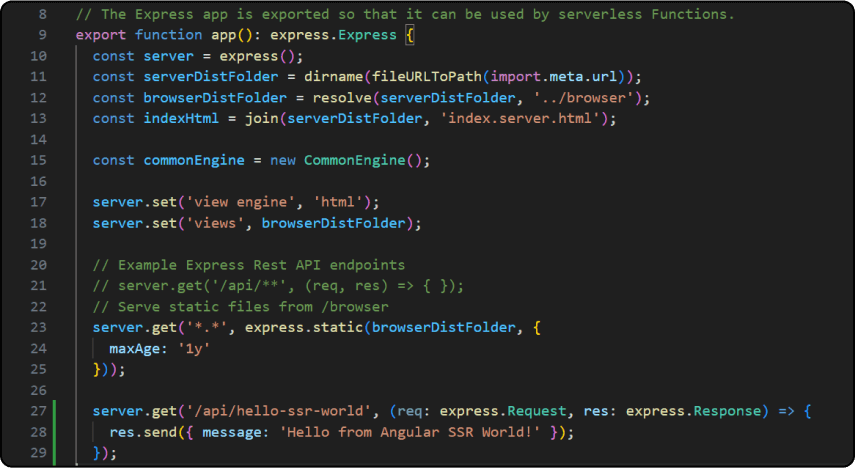
Step 7: Creating and Defining Service
Now, create a service And call the API. As we are running on the same domain so no need to add the prefix URL here.
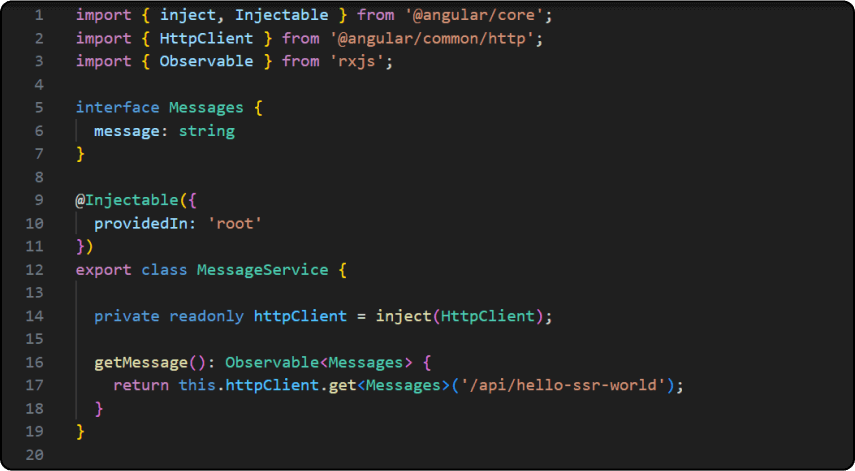
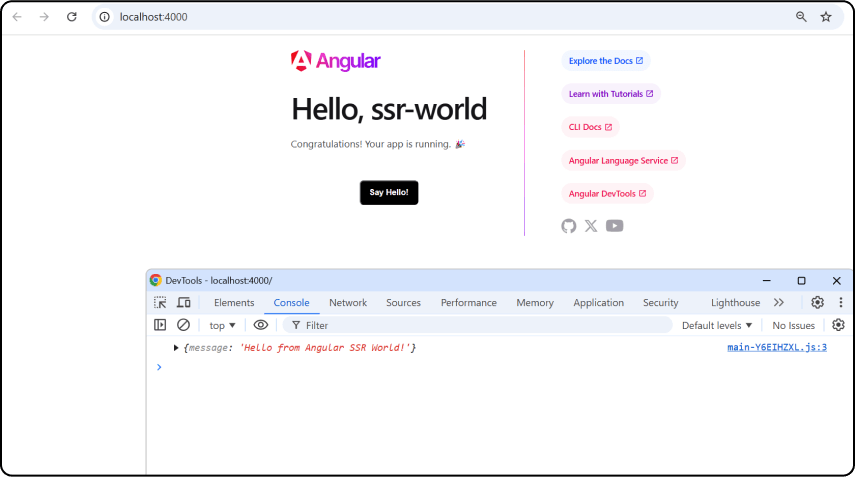
Step 8: Making Interaction With Server APIs
Add a button and invoke the function that will call the service and return the API response(Success/Error).
<button (click)=”printMessage()”>Print Message</button>
And once you click on the button, you can see a message printed in the browser’s console.
Browser Specific API has to Handle While We are Implementing the SSR Project:
Because Angular Server-Side Rendering (SSR) operates on the server rather than the browser, some browser-specific APIs will not function properly when Angular Universal is used with SSR. These browser-specific APIs are dependent on the browser environment and will not work properly in Angular SSR. The following list of browser-specific APIs should be avoided or handled differently in Angular SSR.
When implementing an Angular Server-Side Rendering (SSR) project, it’s essential to handle browser-specific APIs carefully. Since SSR operates on the server rather than the browser, APIs that rely on the browser environment will not function properly. These browser-specific APIs, such as the window, document, and navigator objects, are unavailable during SSR. For instance, the window object, which is crucial for many browser interactions, is only accessible in the browser and is absent in the SSR context. Similarly, APIs like localStorage, sessionStorage, and the navigator object are also browser-dependent and will not work in the server environment. Additionally, services such as Geolocation, WebSockets, Web Workers, and APIs related to cookies, clipboard, and media (like the Audio/Video APIs and Service Workers) require the browser context to operate.
To ensure your SSR application works as intended, these browser-specific APIs need to be avoided or handled differently, such as by implementing fallback mechanisms or using Angular’s platform-specific services to check for the browser environment before making calls to these APIs.
Workaround:
Check for isPlatformBrowser to ensure the code runs only in the browser. Use isPlatformBrowser to conditionally access browser-related properties.
EX, We can add or delete DOM using the isPlatformBrowser functionality. Where we can deal with browser-related code that isn’t going to be rendered on the server side beforehand.

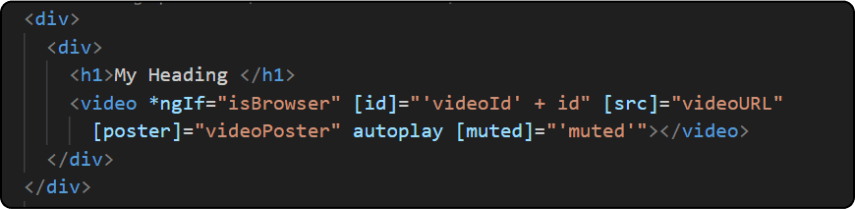
Additionally, You can examine the output of the server-side HTML that Angular Universal renders to see what is rendered during Angular SSR (Server-Side Rendering). Here’s how to use a bash command to accomplish it:

Conclusion:
For any individuals looking to improve user experience, performance, SEO, and load time, Server-Side Rendering (SSR) offers a plethora of advantages. Using Angular SSR with Angular Universal enhances both user and search engine experiences, speeds up initial load times, and significantly improves SEO. Pre-rendering the application’s HTML on the server ensures speedy delivery and complete search engine crawlability. But configuring and maintaining SSR introduces challenges, such as managing server-side state, maximizing efficiency, and ensuring browser APIs function correctly. When properly configured, Angular SSR can significantly enhance the SEO and performance of Angular applications.

Author's Bio:
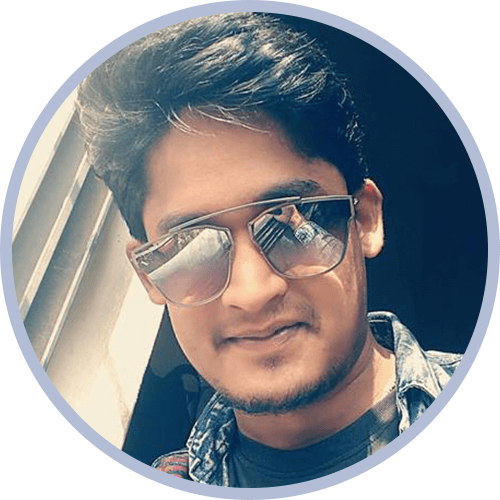
Bharat Kadam has 8 years of experience as a senior software engineer at Mobisoft Infotech with a focus on Angular Frontend development. He is well-versed in Angular, React, Java and contemporary frontend/backend technologies. He is constantly keen to keep ahead of industry trends, is committed to producing clear, maintainable code and resolving challenging issues in the constantly changing frontend/backend environment.