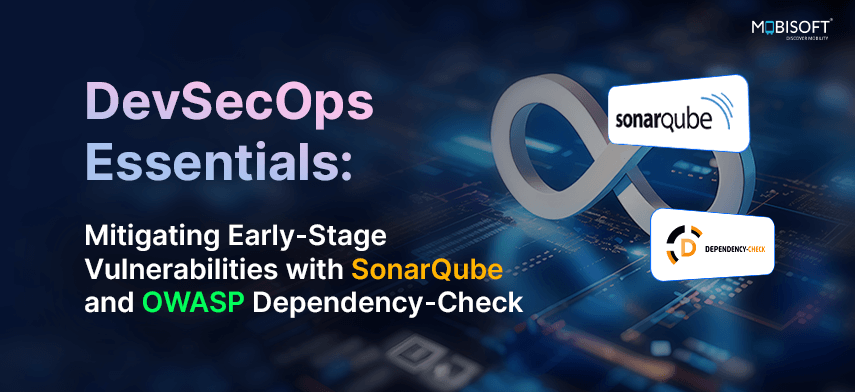
In the ever-evolving world of the internet, effective vulnerability management has become a critical component of the DevSecOps process. As developers strive to create secure applications, it’s essential to integrate software security tools that can identify vulnerabilities and Common Vulnerabilities and Exposures (CVEs) throughout the development lifecycle.
In this article, we will explore various open-source tools, such as SonarQube security and OWASP Dependency-Check, that can be seamlessly integrated at different stages of the development process to enhance code security, specifically focusing on Java applications.
What is Vulnerability?
A vulnerability refers to a weakness in a developed system that can lead to system failure, operational disruptions, data theft, security compromises, data leaks, or breaches. Addressing vulnerabilities is crucial to ensuring the safety and reliability of software systems.
Below are some key factors that can introduce vulnerabilities into your software:
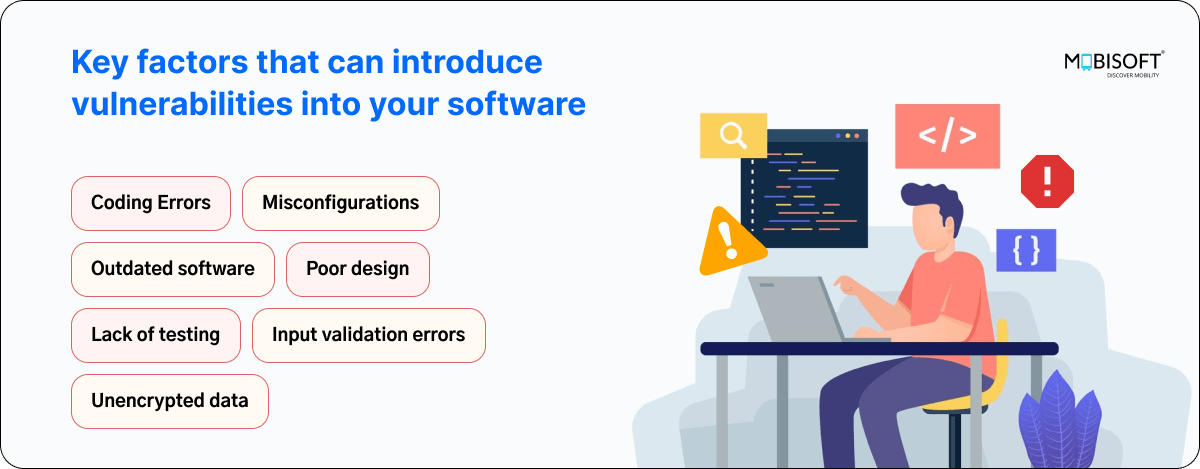
Various tools are available in the market for identifying vulnerabilities and CVEs, but SonarQube security and OWASP Dependency-Check stand out as essential resources for securing applications. These tools can be seamlessly integrated into your development workflow, enhancing your vulnerability management strategy.
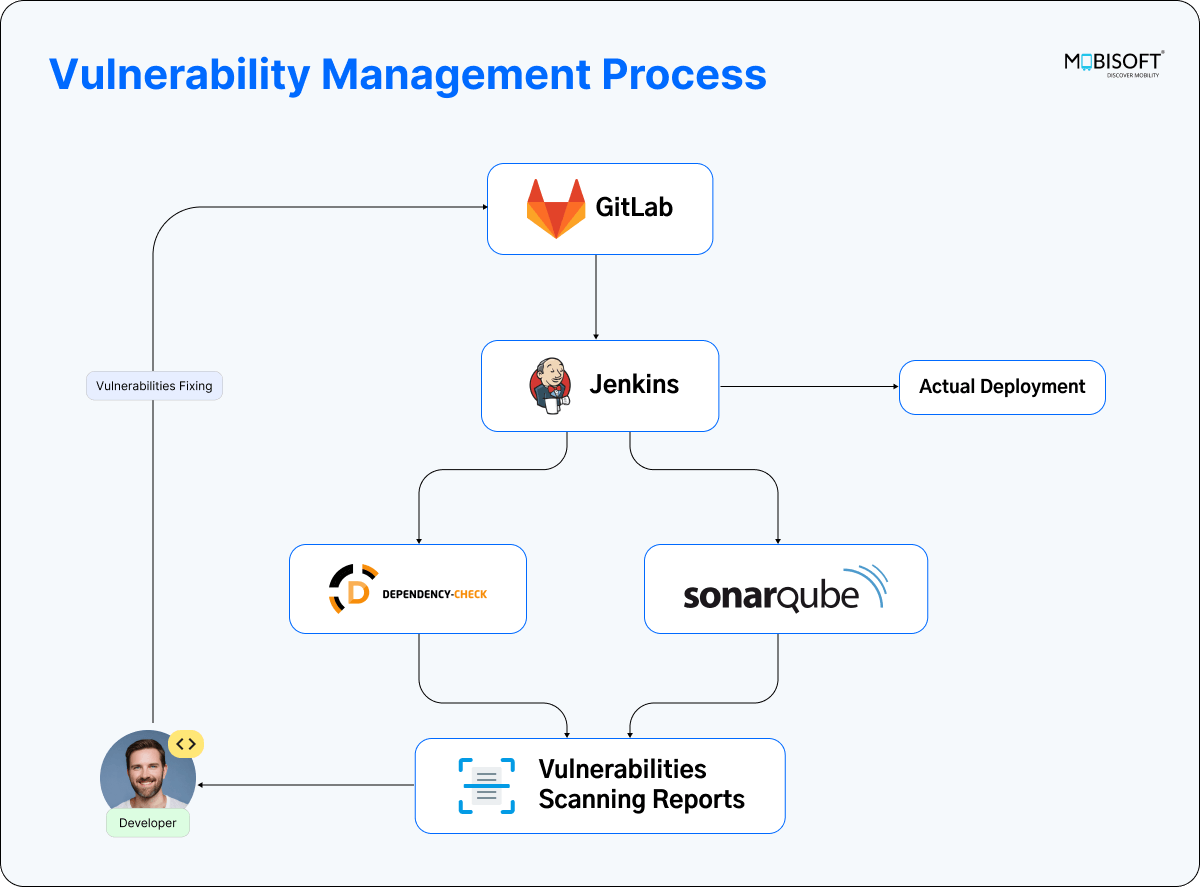
Prerequisites:
- Ubuntu <latest version >
- Install applications ( Java, maven)
- Java Project
- Sonar server ( Hosted )
- Sonar login credentials
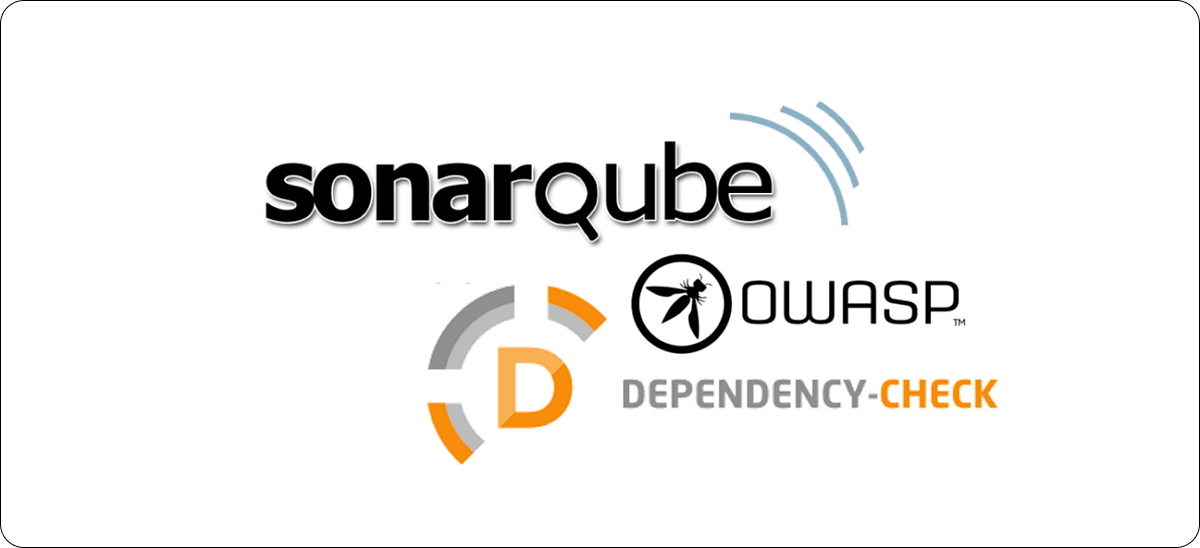
1. SonarScanner:
- This tool is used to assess code quality. It analyzes the code and generates a report based on its findings.
- By integrating seamlessly with your CI pipeline or supported DevOps platforms, it evaluates your code against a comprehensive set of rules. These rules address key attributes such as maintainability, reliability, and security, ensuring thorough analysis for every merge or pull request.
Scanning Capabilities
- Static Code Analysis: Examines source code without execution to identify potential errors and inefficient coding practices.
- Security Analysis: Detects security vulnerabilities such as SQL injection, cross-site scripting (XSS), and buffer overflow risks.
- Concurrency Error Detection: Identifies runtime defects like race conditions, exceptions, resource and memory leaks, and security vulnerabilities.
- Performance Analysis: Monitors software applications during runtime to diagnose and resolve performance bottlenecks.
- Report Generation: Offers comprehensive reporting features to evaluate code quality and effectively identify issues.
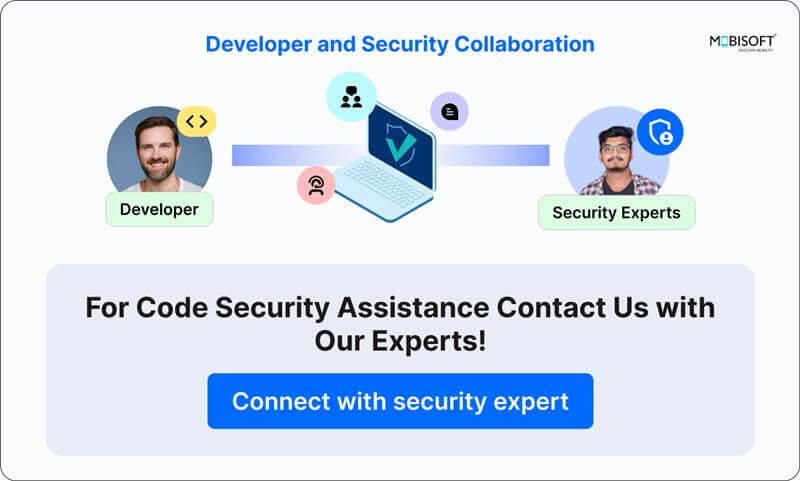
How can we integrate it in Java?
From the local machine
Installation Process for SonarScanner
Install sonar scanner in your machine if not already installed
Step 1: Visit the official SonarSource website to find the latest version of the SonarScanner CLI. Look for the download link for the zip file.
⇒ wget https://binaries.sonarsource.com/Distribution/sonar-scanner-cli/sonar-scanner-cli-4.2.0.1873-linux.zip
⇒ unzip sonar-scanner-cli-4.2.0.1873-linux.zip
Code language: JavaScript (javascript)
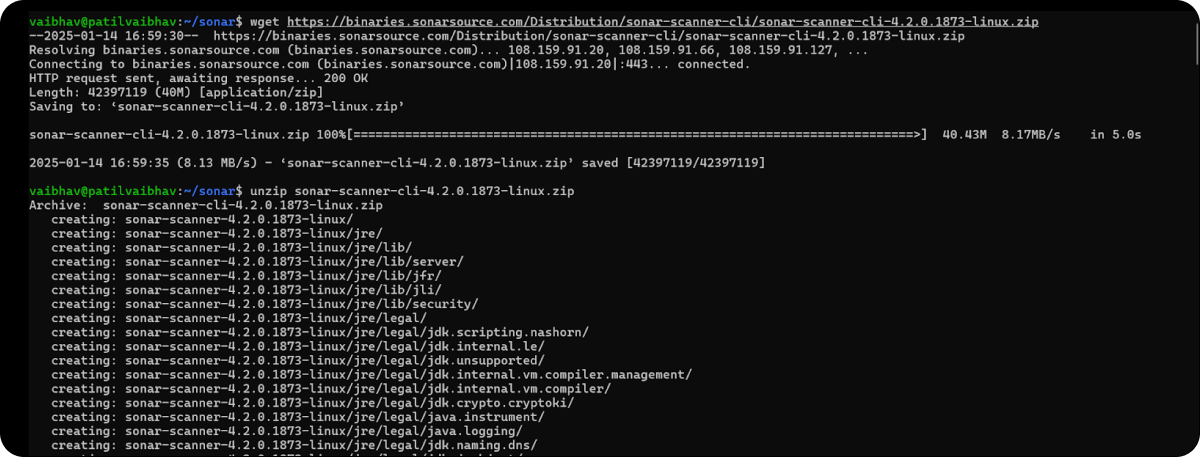
Step 2: To set environment variables for accessing the SonarQube portal from your local machine, execute below commands:
⇒ sudo mv sonar-scanner-4.2.0.1873-linux/ /opt/
⇒ sudo nano /opt/sonar-scanner-4.2.0.1873-linux/conf/sonar-scanner.properties
Code language: JavaScript (javascript)
Add below content in sonar-scanner.properties
sonar.host.url=<your hosted domain for sonar server>
sonar.login=user.name
sonar.password=*****passwd****
Code language: HTML, XML (xml)
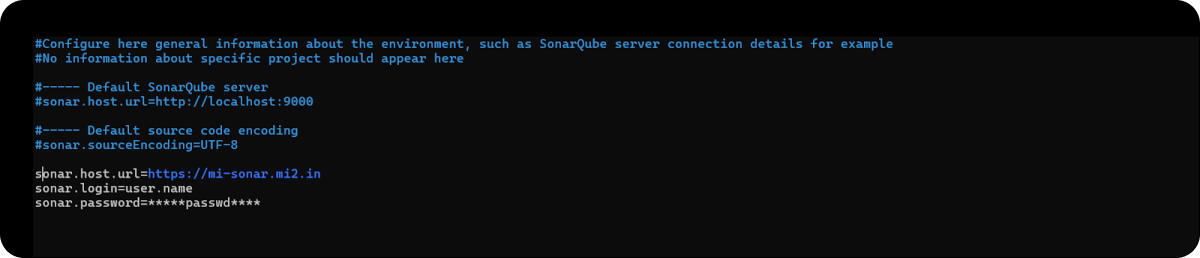
Step 3: To set up a profile on your local machine for accessing SonarQube we need to add sonarpath in sonar-scanner.sh file, for this, execute the below commands
⇒ sudo nano /etc/profile.d/sonar-scanner.sh
##Add path in sonar-scanner.sh file and save the file :
export PATH=”/opt/sonar-scanner-4.2.0.1873-linux/bin:$PATH”
Code language: PHP (php)
Step 4: To apply the changes you’ve made, execute the following command:
⇒ source /etc/profile.d/sonar-scanner.sh
Step 5: To verify Installation of the sonar scanner is completed check the version of it.
⇒ sonar-scanner --version
Step 6: Configure SonarScanner CLI:
- To connect SonarScanner CLI to your SonarQube server or SonarCloud, you need to create and configure a sonar-scanner.properties file in your project root directory.
- Open the sonar-scanner.properties file in a text editor and configure it according to your project requirements. Below is an example configuration:
sonar-scanner.properties
# must be unique in a given SonarQube instance(project_key)
sonar.projectKey=<your_project_key>
# This is the name and version displayed in the SonarQube UI. Was mandatory prior to SonarQube 6.1.
sonar.projectName=<your_project_name>
sonar.projectVersion=0.0.1
# Path is relative to the sonar-project.properties file. Replace "\" by "/" on Windows.
# If not set, SonarQube starts looking for source code from the directory containing
# the sonar-project.properties file.
sonar.sources=src/main/java
sonar.projectBaseDir=.
# Encoding of the source code. Default is default system encoding
sonar.sourceEncoding=UTF-8
sonar.binaries=target/classes/
sonar.java.binaries=target/classes/
Code language: PHP (php)
Step 7: Run SonarScanner CLI
Navigate to your project directory in the Terminal and execute the following command to run SonarScanner CLI:
⇒ sonar-scanner
How can we integrate it into the CICD pipeline?
- To integrate SonarScanner into your CI/CD pipeline, you can create a script that executes SonarScanner commands before building your project.
- In the below script, we are using the Maven plugin; if you want to use sonar-scanner CLI, follow the installation instructions and provide the command in the script in place of Maven.
sonar-scan.sh
#!/bin/bash
# Configuration Variables
SONAR_LOGIN="<sonar_login_user_name>"
SONAR_PASSWORD="sonar_login_pass"
SONAR_HOST="<sonardomain>"
SONAR_SOURCE="src/main/java"
SONAR_BINARIES="target/classes/"
SONAR_JAVA_BINARIES="target/classes/"
SONAR_EXCLUSIONS="**/*DaoImpl.java,**/target/classes/*"
# Run SonarQube analysis
echo "Running SonarQube analysis..."
export _JAVA_OPTIONS="-Xms512m -Xmx1g" # You can set this as per your configuration
# if you are using cli then below part is not required in script just provide the command: sonar-scanner
if mvn clean verify sonar:sonar \
-Dsonar.projectKey=<project_key> \
-Dsonar.host.url="$SONAR_HOST" \
-Dsonar.login="$SONAR_LOGIN" \
-Dsonar.password="$SONAR_PASSWORD" \
-Dsonar.sources="$SONAR_SOURCE" \
-Dsonar.binaries="$SONAR_BINARIES" \
-Dsonar.java.binaries="$SONAR_JAVA_BINARIES" \
-Dsonar.exclusions="$SONAR_EXCLUSIONS"; then
echo "SonarQube analysis completed successfully."
else
echo "SonarQube analysis failed. Please check the errors above."
exit 1
fi
# Change directory back (if necessary)
cd .. || { echo "Failed to change directory."; exit 1; }
Code language: PHP (php)
Add the Script to Your CI/CD Pipeline:
pipeline {
agent any stages {
stage('SonarQube Analysis') {
steps {
script {
sh 'chmod +x sonar-scan.sh' // Make the script executable
sh './sonar-scan.sh' // Execute the SonarScanner script
}
}
}
stage('Build') {
steps {
// Your build commands here (e.g., mvn clean install)
}
}
}
}
Code language: PHP (php)
After executing the SonarScanner in your CI/CD pipeline or on a local machine, log in to the SonarQube / SonarCloud portal to check the status of your code. You should see an overview similar to the screenshot below:
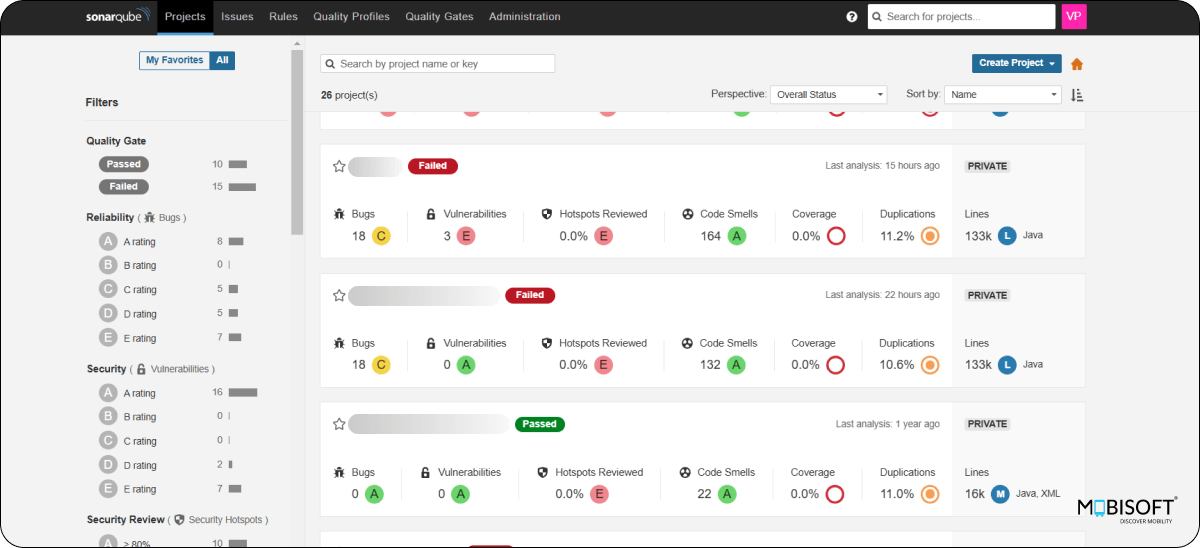
2. OWASP Dependency-Check:
OWASP Dependency-Check is capable of identifying vulnerabilities in web applications, such as compromised authentication, sensitive data exposure, security misconfigurations, SQL injection, cross-site scripting (XSS), insecure deserialization, and the use of components with known vulnerabilities.
Scanning Capabilities–
- Automated Vulnerability Detection: Analyzes application source code and metadata to identify the dependencies used in the project.
- Identifying Dependencies via Common Platform Enumeration (CPE): Detects the CPE identifier associated with each dependency.
- Third-Party Library Detection: Compares CPE identifiers with data from the National Vulnerability Database (NVD) to automatically identify and flag vulnerable libraries used in the application.
- Reporting Results: Generates a detailed report in HTML or XML format, outlining all identified vulnerabilities. The report includes:
- A list of affected libraries and dependencies
- CVE and CPE identifiers
- Severity score metrics
- Links to remediation guidance
How can we integrate it into the code?
We can leverage OWASP Dependency-Check in the code build process with Maven by creating a script and executing it during the CI/CD pipeline.
build.sh
#!/bin/bash
# Step 1: Install dependencies and verify Maven installation
echo "Updating system and verifying Maven installation..."
if mvn -v; then
echo "Maven is installed."
else
echo "Maven is not installed. Please install Maven to proceed."
exit 1
fi
# Step 2: Build the Maven project
echo "Building the Maven project and running OWASP dependency check..."
if mvn -B -s pom.xml -Dmaven.test.skip=true -Pdev clean install org.owasp:dependency-check-maven:aggregate; then
echo "Build successful."
else
echo "Build failed. Please check the errors above."
exit 1
fi
Code language: PHP (php)
Write a Jenkinsfile that incorporates the above script, allowing it to be executed during the CI/CD pipeline:
##
pipeline {
agent any
stages {
stage('Build') {
steps {
script {
sh 'chmod +x build.sh' // Make the script executable
sh './build.sh' // Execute the build script
}
}
}
}
}
Code language: PHP (php)
There is no need to install extra dependencies for the OWASP tool.
After building and executing this tool you will get the report in .html, .XML, and .json format.
By incorporating both SonarQube analysis and OWASP Dependency-Check, we can effectively address a significant number of vulnerabilities and CVEs (Common Vulnerabilities and Exposures) in the code. Below is a Jenkinsfile for a CI/CD pipeline that executes both tools in a single run:
pipeline {
agent any
stages {
stage('SonarQube Analysis') {
steps {
script {
sh 'chmod +x sonar-scan.sh' // Make the script executable
sh './sonar-scan.sh' // Execute the SonarScanner script
}
}
}
stage('Build') {
steps {
script {
sh 'chmod +x build.sh' // Make the script executable
sh './build.sh' // Execute the build script
}
}
}
}
}
Code language: JavaScript (javascript)
Conclusion:
Together, these tools foster a security-focused culture throughout the software development lifecycle, empowering organizations to build robust applications that comply with industry standards and adapt to emerging security threats. By effectively leveraging these resources, developers can greatly enhance their applications’ defenses against malicious attacks, ultimately contributing to a more secure digital environment.

Author's Bio:
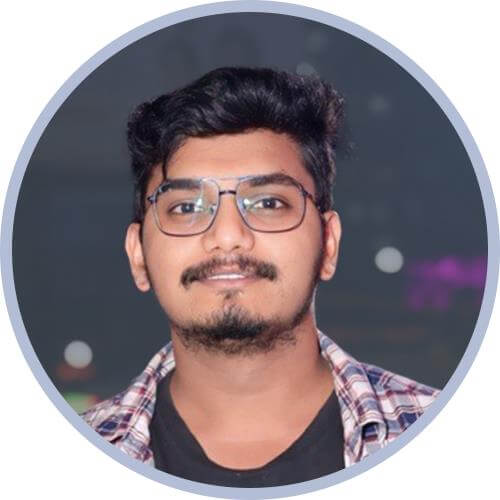
Vaibhav Patil is a seasoned DevSecOps Engineer at Mobisoft Infotech with over 5+ years of expertise in DevOps and cloud infrastructure. He specializes in AWS, GCP, Docker, Kubernetes, CI/CD pipelines and security testing. He excels in designing and maintaining secure,scalable, and cost-efficient systems while ensuring seamless deployments and high availability for web and mobile applications.His dedication to staying at the forefront of technology drives his success in the ever-evolving tech landscape.