A Tab Bar Controller helps to organize an application along functional lines.It is used to switch between multiple pages in an application. Each tab of a tab bar controller interface is associated with a view controller.Whenever a tab is tapped by a user,the tab bar controller object selects the particular tab and displays the corresponding view associated with the tab.This blog will assist you on how to create applications using tab bar controller.
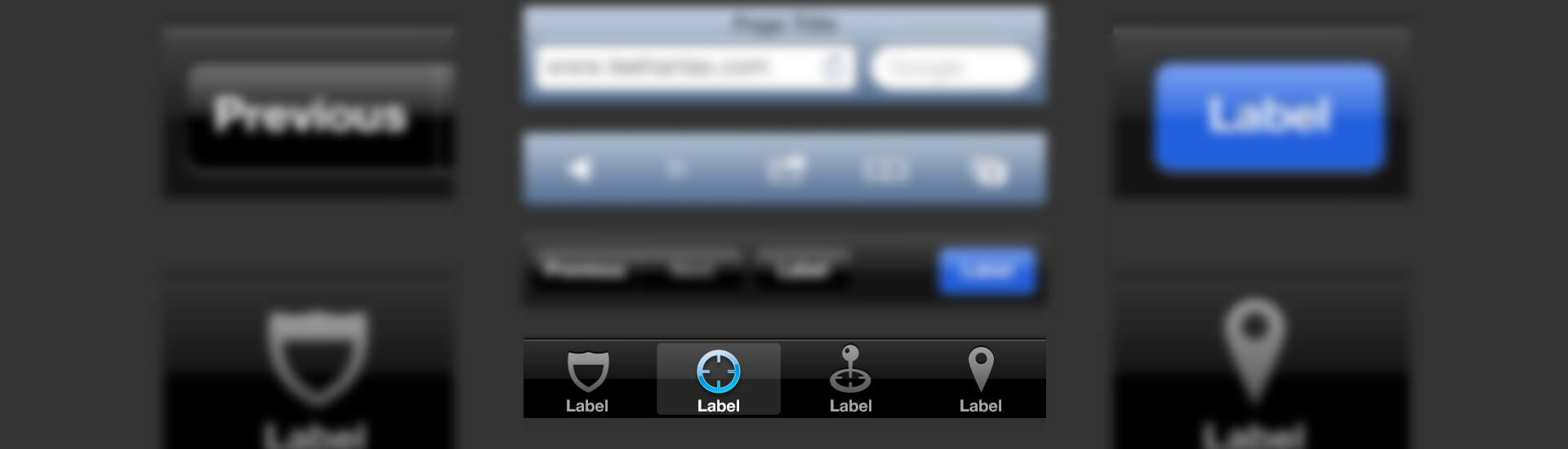
Step 1 : Start Xcode and create a new project by selecting Window- Based Application.
Step 2 : Open “PrjTabBarControllerAppDelegate.h” and put following code in it.
#import <UIKit/UIKit.h> @interface PrjTabBarControllerAppDelegate:NSObject <UIApplicationDelegate> { UIWindow *window; UITabBarController *tcTabBar; } @property (nonatomic, retain) IBOutlet UIWindow *window; @property (nonatomic, retain) IBOutlet *tcTabBar; @endHere ‘”tcTabBar” is an instance variable of type UITabBarController. We have created an outlet, so that we can relate our instance ‘tcTabBar’ with TabBarController.
Step 3 : Open “PrjTabBarControllerAppDelegate.m” and put following code in it. In the ‘applicationDidFinishLaunching’ method we have added the Tab Bar Controller as a sub view to the existing window. Save the project using (command + S).
#import "PrjTabBarControllerAppDelegate.h" @implementation PrjTabBarControllerAppDelegate @synthesize window; @synthesize tcTabBar; - (void)applicationDidFinishLaunching:(UIApplication *)application { // Override point for customization after application launch [window addSubview:tcTabBar.view]; [window makeKeyAndVisible]; } - (void)dealloc { [tcTabBar release]; [window release]; [super dealloc]; } @endStep 4 : Open the Interface Builder by double clicking on MainWindow.xib file. Drag & Drop a Tab Bar Controller from the Library(shift + command + L.). Make sure that the Tab Bar Controller is dropped on the MainWindow.xib since it is not allowed to be placed on the View Controller.We will see a Tab Controller with two bar items created for us. Add one more tab item to it from the library.
As we can see a Tab Bar Item has ‘Title’ & ‘Image’ associated with it. Double Click on Item 1. Open the ‘Attributes Inspector’ (command + 1).In the ‘Atrributes Inspector’ we can set the title & image for Tab Bar Item. The image that we are adding should be present in the Project folder.To add an image to Project right click on ‘Resources’ -> Add -> Existing Files and select the image.
Step 5 : Now connect the Tab Bar Controller to PrjTabBarControllerAppDelegate. Open the ‘Connection Inspector’ (command + 2). Connect “tcTabBar” in the File’s Owner to Tab Bar Controller.
Step 6 : Right click on ‘Classes’ in Group & Files Explorer & add UIViewController subclass file. Select the option ‘With XIB for user interface’. The files added should be equal to number of Tab Bar items present.Here, we add three UIViewController so name them as View1ViewController, View2ViewController, View3ViewController.
Step 7 : Open the View2ViewController.xib by double clicking on it. Set the color of the view from the ‘Attribute Inspector’.Save & Close the .xib file. Similarly set the colours for other views.
Step 8 : Now we need to specify which views should be there in each tab of TabBarController. For this open ‘Interface Builder’. Open ‘Identity Inspector’ (command + 4). Set the class of each Tab Bar Item to its respective view.
Step 9 : Save,Build & Run the project.When we switch the tabs you will observe the views .
You can download the source code here.Output: