Ant Design is a very popular UI component toolkit. It contains almost every UI component that one might require in an Enterprise React application. For styling Ant Design uses Less CSS Preprocessor. Unfortunately the Create React App (CRA) project doesn’t come with inbuilt Less support. In this article we will see how to set up a React project from scratch using CRA with Ant Design and Less support using the craco.
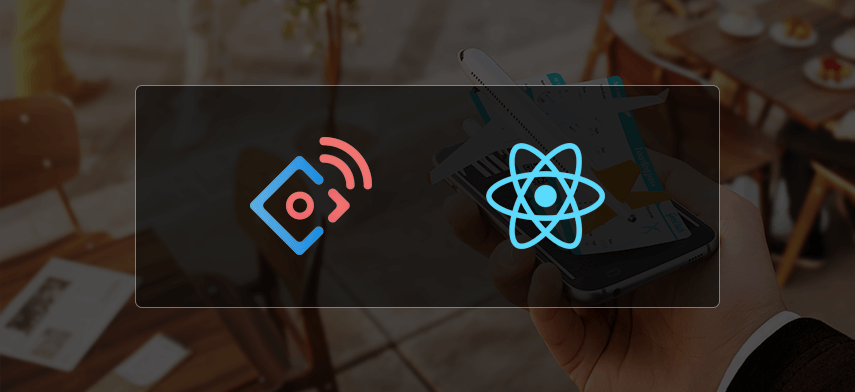
Step 1. Create the Project
npx create-react-app react-sample
cd react-sample
npm start
This will start a browser at: http://localhost:3000/.
If you visit the above URL you should see the default CRA page.
Step 2: Add Ant Design, Craco and Craco Ant Design Plugin
npm install antd @craco/craco craco-antd --save
Once the above mentioned packages are installed, create a new file with name craco.config.js
at the same level as that of package.json
and add the following content to it:
const CracoLessPlugin = require("craco-less");
module.exports = {
plugins: [
{
plugin: CracoLessPlugin,
options: {
lessLoaderOptions: {
modifyVars: {
"@primary-color": "#1DA57A",
"@link-color": "#1DA57A",
"@border-radius-base": "2px"
},
javascriptEnabled: true
}
}
}
]
};
In this file pay special attention to the modifyVars
section. This section allows you to override the Less variables specified by the Ant Design project. In the above example we have replaced the default blue primary color that Ant Design uses with a shade of green.
Next we need to change the default scripts in the package.json
file. Change the scripts section in your package.json
with the following:
"scripts": {
"start": "craco start",
"build": "craco build",
"test": "craco test",
"eject": "react-scripts eject"
},
Step 3: Rename the App.css
file to App.less
To use Less in your project, all you need to do is to use .less
extension instead of the .css
extension, craco will take care of the rest!
Rename the App.css
file to App.less
. Also replace it’s contents with the following:
@import '../node_modules/antd/lib/style/index';
.App {
padding: 20px;
h1 {
color: @heading-color
}
}
Step 4: Modify App.js
to use Ant Design
Replace the contents of the App.js
file with the following:
import React, { Component } from 'react';
import { Button } from 'antd';
import 'antd/lib/button/style';
import './App.less';
class App extends Component {
render() {
return (
<div className="App">
<h1>Create React Project with Ant Design and Less Support</h1>
<Button type="primary">Button</Button>
</div>
);
}
}
export default App;
Here we are just using a Ant Design button along with the h1
tag. In the App.less
file above, we have used the @heading-color
Ant Design variable to specify the color for the h1
tag.
Also it’s important to notice, that we are importing the individual button component styles with import 'antd/lib/button/style';
instead of importing the whole Ant Design css. This helps us to keep the final bundle size as small as possible.
That’s it! We are done. To test our project run:
npm start
and point your browser to: http://localhost:3000/
Just to ensure that you are using Less through out the project, I will suggest that you should rename the index.css
file to index.less
and modify the index.js
file accordingly.
Hope this tutorial helped you to setup a React Project from scratch using Ant Design and Less Support. You can download the source code for this project from here.

Author's Bio:
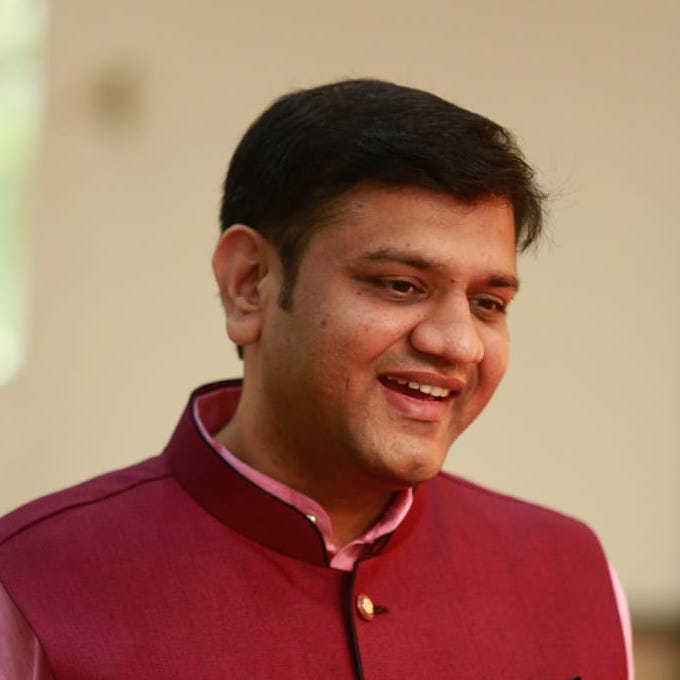
Pritam Barhate, with an experience of 14+ years in technology, heads Technology Innovation at Mobisoft Infotech. He has a rich experience in design and development. He has been a consultant for a variety of industries and startups. At Mobisoft Infotech, he primarily focuses on technology resources and develops the most advanced solutions.