Being android application developer, one may come across losing variables data which may have arisen when system kills your activity when it is in background or currently not visible. While designing android app it is very important to consider whole life cycle of application. Sometimes there are chances to miss out any of the state of the activity life cycle.
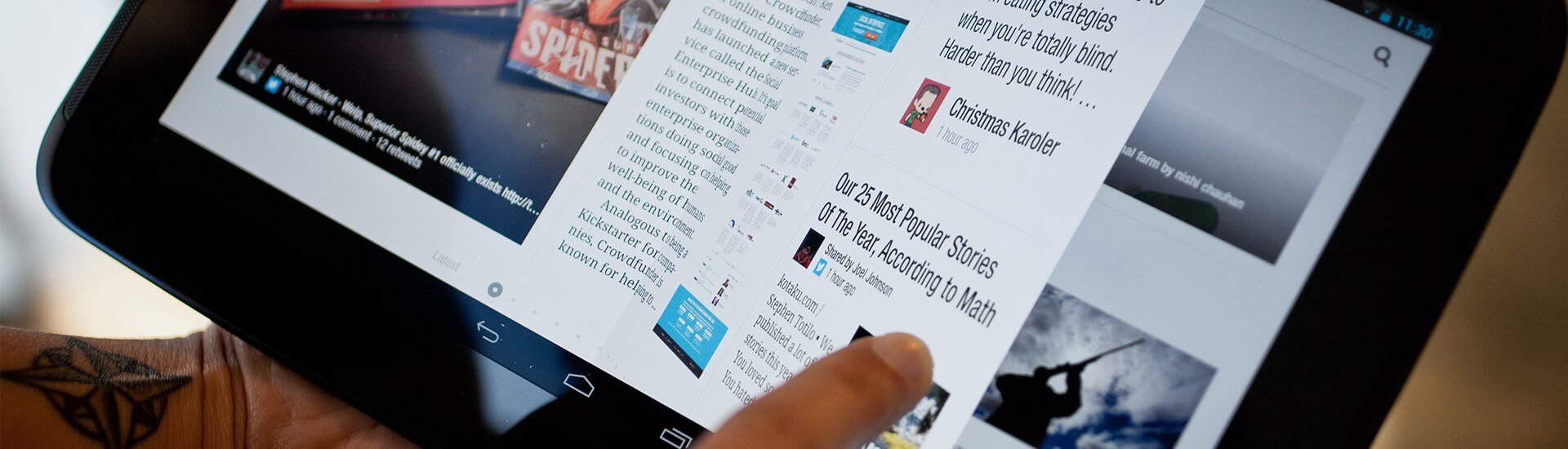
System killed activity is one of the crucial state which can lead to unexpected behavior of app if not given attention at the time of development. Android system can kill activity of application which is currently not visible or which is in background when other visible or any priority process needs room in device memory. In such situation if developer has used some global variables which he has referred in many other activities, he can end up in losing values of those global variables when activity comes to foreground because activity where variables are instanciated has been already killed by system.
Instead of declaring such global variables in one activity and referring in all other activities, one can create single instance class which is extended from Application class.
About Application class
Application class is the base class where you can maintain global variables. One can override Application class by extending his own class from it and specifying that class in AndroidManifest.xml’s tag.
Application class exists through out the app life cycle. Creating a static singletons can serve the purpose instead of extending and overriding Application class. But if one needs to manage other memory leaks he can override Applications class methods.
Our own class which is extended from Application class is as follows:
[java] import android.app.Application; public class GlobalApplication extends Application { public String helloFromGlobalApplication = "Hello From GlobalApplication"; private static GlobalApplication singleton; public static GlobalApplication getInstance() { return singleton; } @Override public void onCreate() { super.onCreate(); singleton = this; } } [/java]
Then need to add this newly created class in app manifest file as follows
[xml] <application android:name="<your package name>.GlobalApplication"> ........ ........ </application> [/xml]
Now when you run your app, your application/package will be created and at the same time your newly created GlobalApplication class would be instanciated. Now you can use helloFromGlobalApplication variable from GlobalApplication anywhere in other activities as follows.
[java] public class MainActivity extends Activity { @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); Log.d("helloFromGlobalApplication : ", GlobalApplication.getInstance().helloFromGlobalApplication); } } [/java]
So extending Application class helps you to create single place for global instanciation for those data or variables which you need through out the app.