There’s a lot of new syntax in Swift, so we thought it might be useful if we put together a cheat sheet/quick reference on this particular language. Without further ado, let’s see what are they:
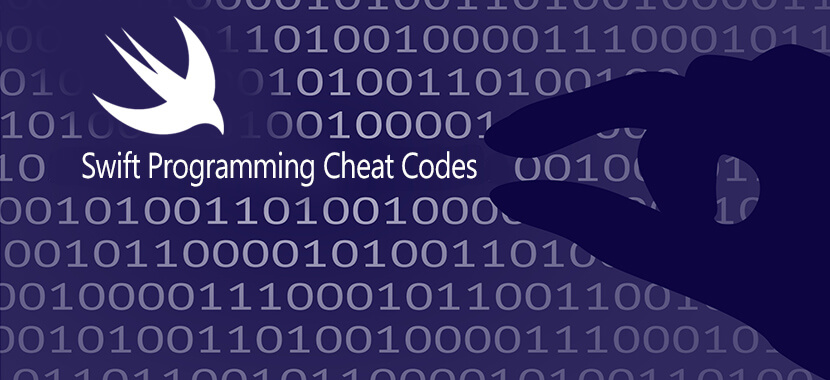
1. No .h and .m file, only one file with .swift extension
2. No semicolon at the end of the statement
3. No @ for literal strings
4. No pointers. So, no use of asterisk
5. Cannot use ’nil’ with non-optional variables or constants
6. No Mutable or Immutable concepts, var use to declare mutable and let use to declare immutable data type.
7. In swift, strings are value type not object type.
8. Constants declared as ‘let’ and variables with ‘var’
9. Declaration and initialisation i.e. var message. For example, String= “Hello”
10. Multiple declaration i.e. var red, green,blue i.e. Double
11. Print to console i.e. println(“Print”)
12. String interpolation i.e. \(variable_name)”)
13. No parenthesis for if i.e. if age < 60
14. Assertion i.e. assert(age >= 0, “A person’s age cannot be less than zero”)
15. Compound assignment operators i.e. +=
16. Unary minus operator i.e. number
17. Arithmetic i.e. 2+3
18. Reminder operators i.e. 2%3
19. Comparison operators i.e. a==b
20. Overflow operators i.e. &+
21. Nil coalescing operators i.e. a ?? b
22. Range operator i.e. 1…5
23. Half open rage operator i.e. 1..
24. Logical operators i.e. a || b
25. String Concatenation i.e. string3 = string1 + string2 or string.append(“string”)
26. Array declaration & initialication i.e. var shppinglist i.e. [String] = [“Eggs”,“milk”]
27. Set value of element in array i.e. set value i.e. shoppingList[0] = “Baking powder”
28. Get value from array i.e. var value = shoppingList[0]
29. Change range of value of an array i.e. shoppingList[0…2] = [“Apple”,”Blackberry”]
30. Array has functions like i.e. iEmpty, Append,InsertAtIndex,RemoveAtIndex, RemoveLast
31. Array Iteration 1, for example,
for (index, value) in enumerate(shoppingList) { statements }
32. Array Iteration 2, for example,
for index in 1…5 { statements }
33. Array Iteration 3, for example
for _ index in 1…5 { statements }
34. Array Iteration 4, for example
for var index = 0; index < 5; ++index { statements }
35. Dictionary declaration and initialisation i.e. var nameDict i.e.[string, string] = [“FirstName” – “nam”, “LastName” – “lastname”]
36. Set value in dictionary i.e nameDict[“FirstName”] = “Mahesh”
37. Get value in dictionary i.e var firstName = nameDict[“FirstName”]
38. Dictionary has functions like isEmpty, count, updateValue(_,Forkey) ,removeValueForKey, keys,values
39. Iteration with dictionary like
for (key,value) in nameDict { statements }
40. Empty Dictionary initialisation i.e. var dict = [Int, String] ()
41. While loop :
while condition { statements }
42. Do-while loop :
Do { statements } while condition
43. If -else :
if condition { statements } else { statements }
44. Switch :
1. switch ‘value’ { case value1 : ’statements' case value2 : ’statements’ Default: ’Statements’ }
2. No implicit fall through
3. Tuples can be used as case value in switch
4. Control transfer statements are also available like break, continue & fall through
45. Function i.e.
Keyword FunctionName Parameter:DataType Return type func sayHello:(helloInput:String) ->String { return stringValue }
46. Variadic function
func arithmeticMean (numbers: Double...) -> Double { var total: Double = 0 for number in numbers { total += number } return total / Double(numbers.count) }
47. Functions with multiple parameters Extra info about parameter Extra info about parameter
func sayHello(to person: String, and anotherPerson: String) -> String { return "Hello \(person) and \(anotherPerson)!" }
48. Call by reference with inout and & operator
var arr = [1, 2, 3] func addItem(inout localArr: [Int]) { localArr.append(4) } addItem(&arr)
49. Function with return type as Tuple
func minMax(array: [Int]) -> (min: Int, max: Int) { var min var max return (currentMin, currentMax) }
50. Nested functions :
func chooseStepFunction(backwards: Bool) -> (Int) -> Int { func stepForward(input: Int) -> Int { return input + 1 } func stepBackward(input: Int) -> Int { return input - 1 } return backwards ? stepBackward : stepForward }
51. Closure declaration :
Structure { (parameters) -> return type in statements } Example { (s1:string,s2:String) -> Bool in return s1 > s2 }
52. Enumeration declaration :
enum name { case 1 case 2 case 3 }
53. Enumeration with associated values :
enum barcode { case UPCA(Int, Int, Int, Int) case QRCode(String) }
54. Strings, Array, Dictionaries, Structures are value types and Classes are reference types
55. “override” is the keyword used to override observers, getter/setter, functions or subscript
56. “final” is the keyword used to stop overriding
57. External name means “user-defined-name” is used to differentiate the definition of multiple init method with single parameter
58. Class getting Properties from base must be initialised
59. In case of optional chaining use “?” to unwrap data, Instead of using “!”
60. For down casting a reference type variables use “as?” & “as!”. While for value type use “as” keyword
Hope the aforementioned cheat sheet & references were insightful for all the Swift programmers.
Written by –
Mahesh Asabe
Tech Lead – Mobisoft Infotech