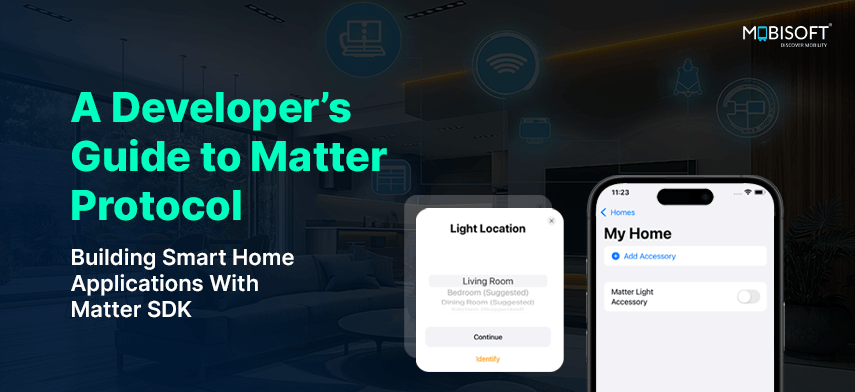
The evolution of smart homes has fundamentally changed how we engage with technology in our daily lives. From voice-activated assistants to automated lighting and security systems, the opportunities seem endless. Yet, one of the biggest challenges developers face in this rapidly expanding space is ensuring interoperability—the ability for diverse smart devices to work together seamlessly, regardless of brand or platform.
What is Matter, and Why Is It Important for Smart Home Protocols?
Matter is a new, open-source standard for smart home devices that aims to make everything in your connected home work together—no matter the brand or platform. Think of it as a universal language for all your smart gadgets, whether it’s your smart lights, thermostats, locks, or security cameras.
Before Matter, it was like a tech version of a frustrating puzzle. You might have an Alexa speaker, a Google Nest thermostat, and smart bulbs from another brand, but they didn’t always play nice with each other. That’s because each brand used its own system, and it was a real hassle to get them all connected. Matter changes that.
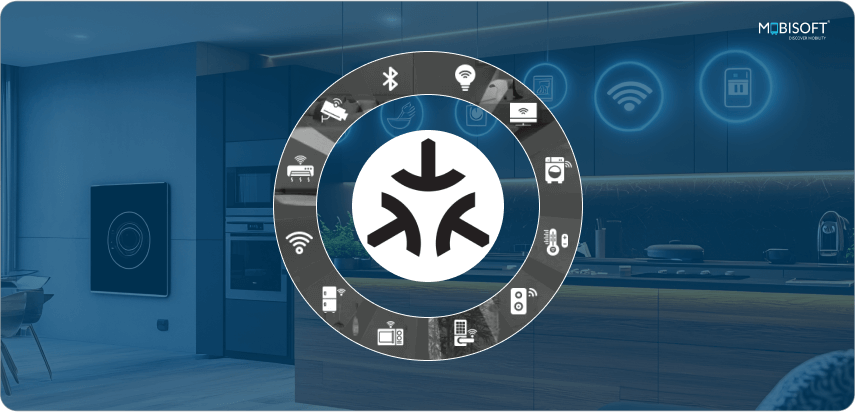
Why Matter Is So Important for Developers and Smart Home Connectivity
1. Everything Works Together: Matter makes sure that no matter which brand you choose, your smart devices can talk to each other. Whether it’s an Apple, Amazon, or Google device, they’ll all work seamlessly in your home.
2. Better Security: With Matter, security is built right into the system. Your devices will encrypt your data and keep everything safe, which is a huge relief when you’re dealing with devices that control things like your door locks or cameras.
3. Easier for Developers: For developers, Matter means they can create one app or solution that works across all major platforms. No more reinventing the wheel for each system (like Google Home, Apple HomeKit, or Alexa). It’s more efficient and saves a lot of time.
4. Ready for the Future: Big companies like Apple, Google, Amazon, and others are backing Matter, which means it’s designed to keep up with new technologies and trends. It’s built to support future devices, so your home won’t get outdated anytime soon.
5. Peace of Mind for Consumers: For everyday people, Matter ensures you can buy new smart gadgets with confidence. You won’t need to worry about whether they’ll work with your existing setup. Everything will just sync up, now and in the future.
In a nutshell, Matter is here to make our lives easier and smarter by bringing all the tech in our homes into one cohesive, secure, and future-ready system. It’s a game-changer for both developers and consumers, ensuring everything works together smoothly and securely.
This guide will walk you through the process of adding Matter devices to your iOS app, providing you with the tools to build smart home solutions that are secure, interoperable, and future-ready.
Get ready to dive into the world of smart home integration! We’ll be using HomeKit to seamlessly add Matter devices as accessories to our iOS app. Apple recommends HomeKit as the best solution for integrating Matter devices into the iOS ecosystem. Serving as the central hub for Matter on Apple devices, HomeKit provides a smooth and secure way to manage these devices. It handles all the essential tasks, including device authentication, secure key exchanges, network details sharing, and device setup.
By utilizing HomeKit, we’re also future-proofing our app. Apple ensures that HomeKit stays aligned with the latest Matter standards, iOS updates, new device types, and security improvements, keeping our app compatible and up-to-date.

Configuring a Matter Device Simulator Using the CHIP Tool for Smart Home Development
Let’s dive into the process of configuring the Matter device simulator using the CHIP (Connected Home Over IP) Tool.
Prerequisites and Steps to Run a Matter Device Simulator:
Step 1: System Requirements
For macOS, install the following prerequisites:
brew install openssl
brew install pkg-config
brew install ninja
brew install git
brew install gcc
brew install python3
Step 2: Setting Up the Development Environment
- Clone the Matter repository:
git clone https://github.com/project-chip/connectedhomeip.git
- Navigate to the repository directory:
cd connectedhomeip
- Update the submodules:
git submodule update --init
Step 3: Build Environment Setup
- Bootstrap the environment:
sh ./scripts/bootstrap.sh
- Activate the environment:
source scripts/activate.sh
Note: Grant execute permission to make the directory searchable/accessible
chmod +x scripts
Step 4: Build the Lightning App
Execute the following command in your terminal to build the lighting app:
./scripts/examples/gn_build_example.sh examples/lighting-app/linux out/lighting-app
Note: After executing this command, the output directory should be structured as shown below:
connectedhomeip/
├── out/
│ └── lighting-app/
│ └── chip-lighting-app
├── scripts/
├── examples/
└── src/
Step 5: Verify the Network
Check your network interfaces:
ifconfig
- For WiFi, it is typically
en0
on Mac. - For Ethernet, it might be
en1
or another interface.
Step 6: Running the Matter Lighting Simulator
Execute the following command to start the Matter lighting simulator:
./out/lighting-app/chip-lighting-app --wifi --interface en0
Command Breakdown:
./out/lighting-app/chip-lighting-app
: The executable for the Matter lighting device simulator.--wifi
: Instructs the simulator to use WiFi for networking.--interface en0
: Specifies the WiFi network interface (typically en0 for WiFi on macOS).
Purpose:
- Simulates a Matter lighting device on your local network.
- Makes the device discoverable by HomeKit.
- Allows the device to be added to your Home app as a real Matter device.
Note:
- Ensure you’re in the
connectedhomeip
directory when running this command. en0
is generally the WiFi interface on Mac; verify your interface name using theifconfig
command.- The simulator must be running before attempting to add it to your app.
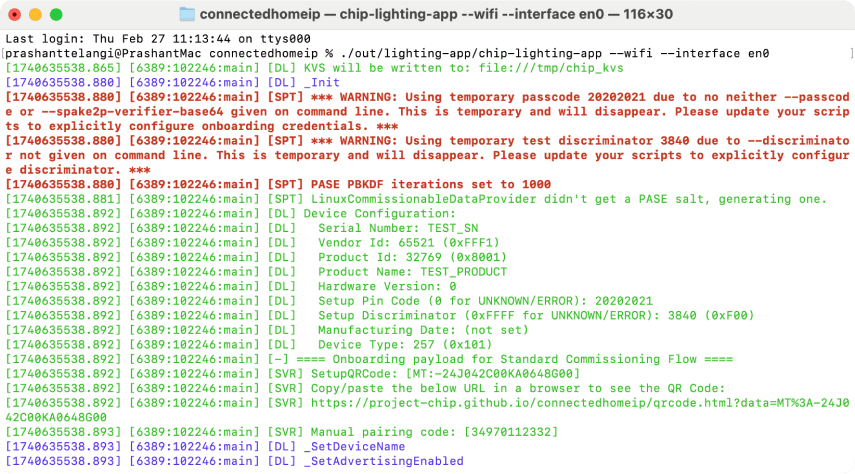
Voila! Our Matter device simulator is now up and running on the network. To add the Matter device via HomeKit, simply scan the QR code. You can find the URL in the console log.

After opening the URL in your browser, you should see a QR code.
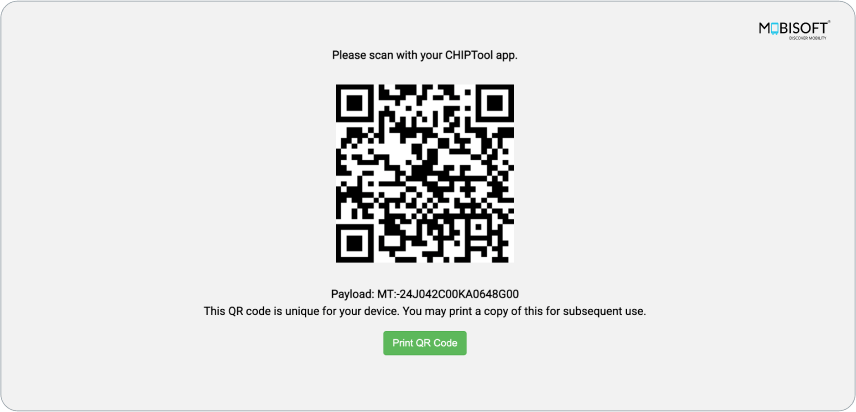
Common Issues and Solutions
1. Build Failures:
If the build fails, try the following steps:
- Clean the Build:
rm -rf out/lighting-app
- Rebuild with Debugging:
./scripts/examples/gn_build_example.sh examples/lighting-app/linux out/lighting-app --debug
2. Unable to Add Accessory After Matter Device is Running:
If you’re unable to add an accessory after your Matter device is running, follow these steps:
- Terminate the Existing Simulator:
killall chip-lighting-app
- Rebuild the Simulator:
./out/lighting-app/chip-lighting-app –wifi –interface en0
Configuring and setting up your iOS application to add a Matter device through HomeKit.
Step 1: Register your bundle ID and enable the capabilities listed below in both your bundle ID settings and your Xcode project.
- HomeKit
- Access Wi-Fi Information
- Matter Allow Setup Payload
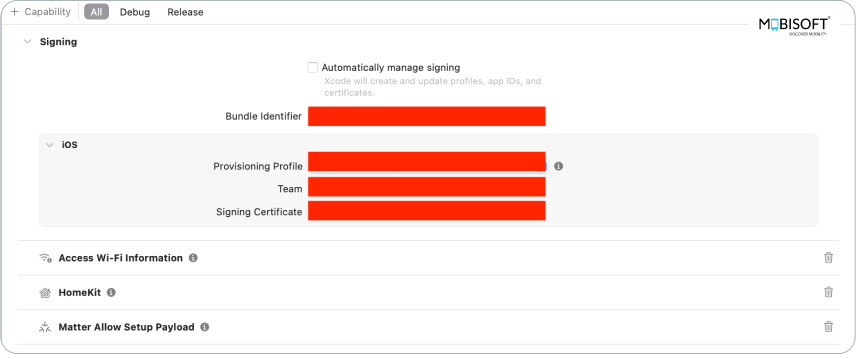
Step 2: Add required Info.plist entries
<!-- Home Kit -->
<key>NSHomeKitUsageDescription</key>
<string>This app needs access to HomeKit to control your Matter devices</string>
<!-- Local Network -->
<key>NSLocalNetworkUsageDescription</key>
<string>This app needs access to discover and control Matter devices on your local network</string>
<!-- Bonjour Services -->
<key>NSBonjourServices</key>
<array>
<string>_matter._tcp</string>
<string>_matterc._udp</string>
</array>
Code language: HTML, XML (xml)
Note: iOS Development Prerequisites:
- Xcode 14.0 or later
- iOS 16.1 or later on the test device
- Apple Developer account
Step 3: Setting Up a New Home using the HomeKit SDK in iOS
class MatterManager: NSObject {
static let shared = MatterManager()
let homeManager = HMHomeManager()
var homesUpdateCallback: (() -> Void)?
private override init() {
super.init()
homeManager.delegate = self
}
func createHome(named name: String, completion: @escaping (Result<Void, Error>) -> Void) {
homeManager.addHome(withName: name) { [weak self] home, error in
if let error = error {
completion(.failure(error))
} else {
completion(.success(()))
self?.homesUpdateCallback?()
}
}
}
...
}
Code language: HTML, XML (xml)
- MatterManager is a Singleton class that can be accessed using MatterManager.shared.
- HomeKit Manager:
let homeManager = HMHomeManager()
This creates an instance of Apple’s HomeKit manager, which oversees the management of homes, rooms, and accessories.
- Create Home Method:
func createHome(named name: String, completion: @escaping (Result<Void, Error>) -> Void) {
homeManager.addHome(withName: name) { [weak self] home, error in
...
}
}
Code language: JavaScript (javascript)
Inside the function, we are calling homeManager.addHome(withName: name) from HMHomeManager. This method is responsible for adding a new home to the HomeKit setup, using the provided name.
Step 4: Integrating a Matter Device as an Accessory into the Home
class MatterManager: NSObject {
...
private let setupManager = HMAccessorySetupManager()
...
func addAccessory(to home: HMHome) async throws {
let setupManager = HMAccessorySetupManager()
let request = HMAccessorySetupRequest()
// Configure the request for the home
request.homeUniqueIdentifier = home.uniqueIdentifier
// Perform the setup
do {
let result = try await setupManager.performAccessorySetup(using: request)
print("Accessory setup completed successfully: \(result)")
homesUpdateCallback?()
} catch {
print("Failed to setup accessory: \(error)")
throw error
}
}
...
}
The HMAccessorySetupManager
is responsible for commissioning Matter devices.
In this function, we create an HMAccessorySetupRequest
and call the performAccessorySetup
method, which adds the Matter device to the Home.
The process is asynchronous because it performs the following tasks:
- Discovers the device on the network
- Handles security handshakes
- Transfers network credentials
- Completes the Matter commissioning process
Note: Before adding the accessory, ensure that your Matter Device simulator is running. Please refer to the steps in the “Configuring a Matter Device Simulator using the CHIP Tool” guide for setup.
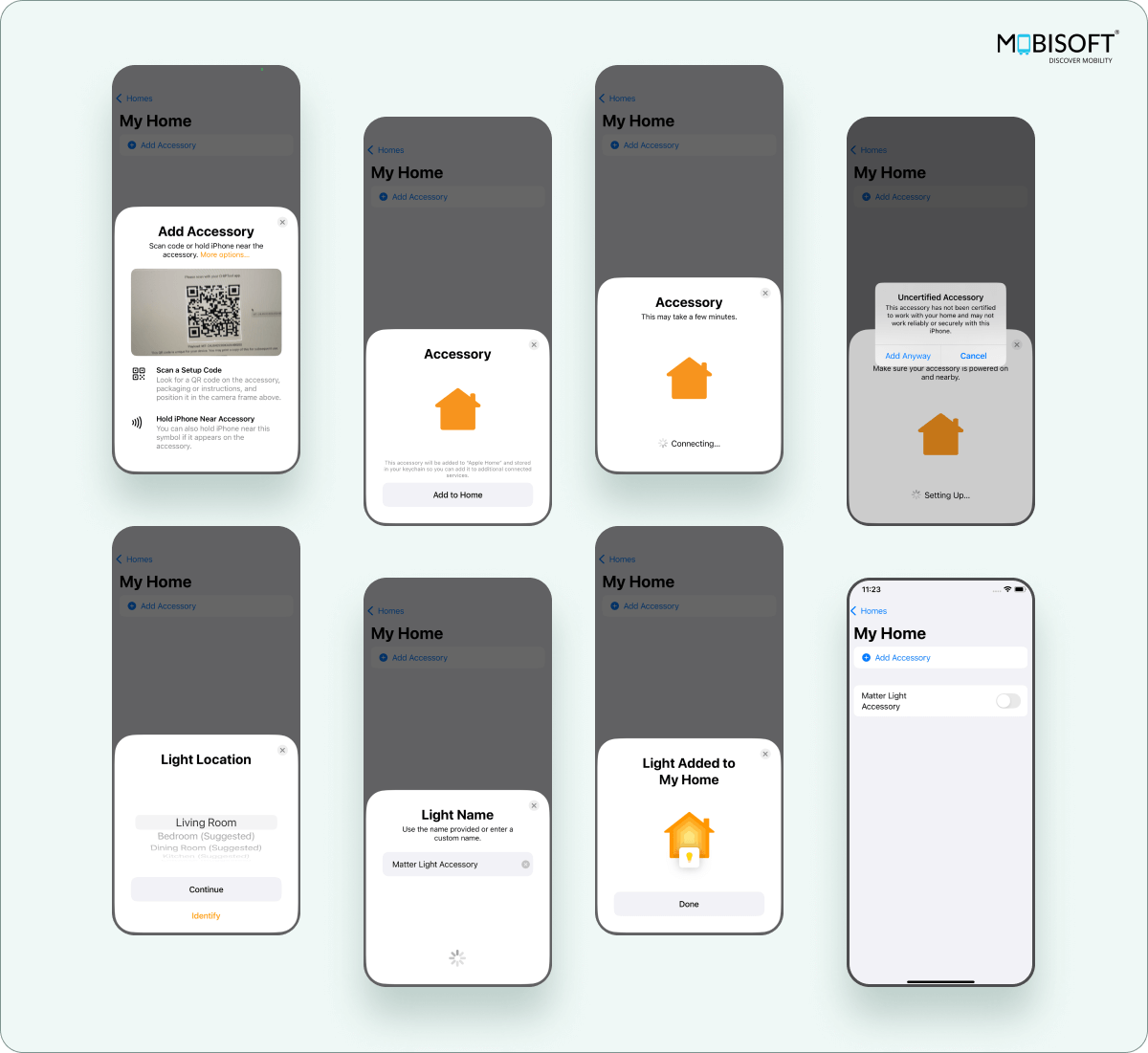
The Matter device should now be visible as an accessory in your Home. You can also verify it within the Home app, where you’ll see the accessory added to your Home.
Step 5: Controlling Matter Device Accessory.
func toggleLight(_ accessory: HMAccessory) async throws {
guard let lightService = accessory.services.first(where: { service in
service.serviceType == HMServiceTypeLightbulb
}) else {
throw HMError(.invalidParameter)
}
guard let powerCharacteristic = lightService.characteristics.first(where: { characteristic in
characteristic.characteristicType == HMCharacteristicTypePowerState
}) else {
throw HMError(.invalidParameter)
}
// Toggle the current state
let currentValue = powerCharacteristic.value as? Bool ?? false
try await powerCharacteristic.writeValue(!currentValue)
}<span style="font-weight: 400;"> </span>
Code language: JavaScript (javascript)
toggleLight
function handles controlling HomeKit-enabled light bulbs.- It searches for a light bulb service in the accessory’s services array. It looks specifically for services of type HMServiceTypeLightbulb.
- Then, it looks for the power state characteristic within that light service. This characteristic represents the on/off state of the light bulb.
- Finally, it performs the actual toggle: Reads the current power state (defaults to false if the value can’t be read as a boolean). Inverts that state using the ! operator
When the light is turned ON, you should see the console log in your terminal displaying Toggle on/off from state 0 to 1
.
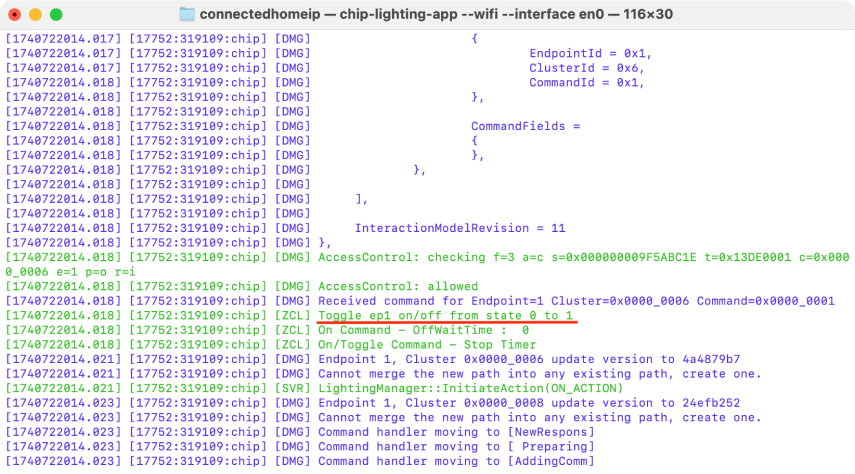
Toggle on/off from state 0 to 1:
0 = OFF state
1 = ON state
The device is switching from OFF to ON
When the light is turned OFF, you should see the console log in your terminal displaying Toggle on/off from state 1 to 0.
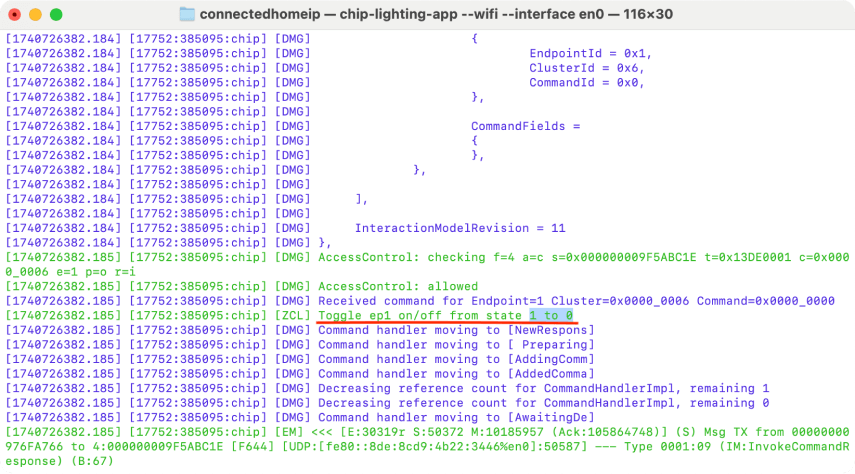
Toggle on/off from state 1 to 0:
0 = OFF state
1 = ON state
The device is switching from ON to OFF
Summing It Up
I hope you found configuring the Matter device with your iOS app via HomeKit both enjoyable and useful. This integration provides a smooth way to control your smart devices directly from your iOS app, enhancing your smart home experience. If you’d like to explore the code further or customize it for your own setup, you can download it from here.

Author's Bio
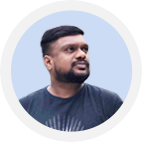
Prashant Telangi brings over 15 years of experience in Mobile Technology, He is currently serving as Head of Technology, Mobile at Mobisoft Infotech. With a proven history in IT and services, he is a skilled, passionate developer specializing in Mobile Applications. His strong engineering background underscores his commitment to crafting innovative solutions in the ever-evolving tech landscape.