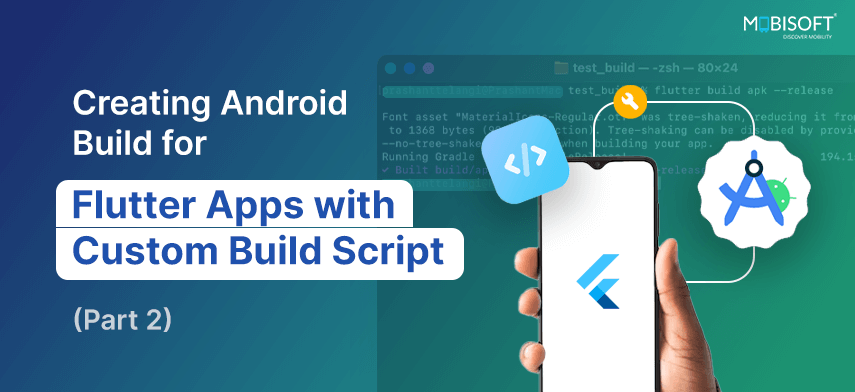
In Part 1, we focused on writing custom build scripts for an iOS app developed using Flutter. These scripts were designed to automate the process of generating the IPA (iOS App Store Package) file, which is essential for distributing the app on iOS devices. In Part 2, we will shift our focus to Android, where we will explore the process of creating build scripts for Android apps using Flutter that automate the generation of the APK (Android Package Kit) file. This will involve setting up the necessary Flutter build configuration and understanding the tools that Flutter provides to streamline the Flutter Android build process.
Creating Android Build for Flutter Apps
Step 1: Let’s build the Android project manually using the Flutter command.
Open Terminal, navigate to your Flutter project directory, and execute the following command:
flutter build apk --release
The command above should successfully generate the APK.
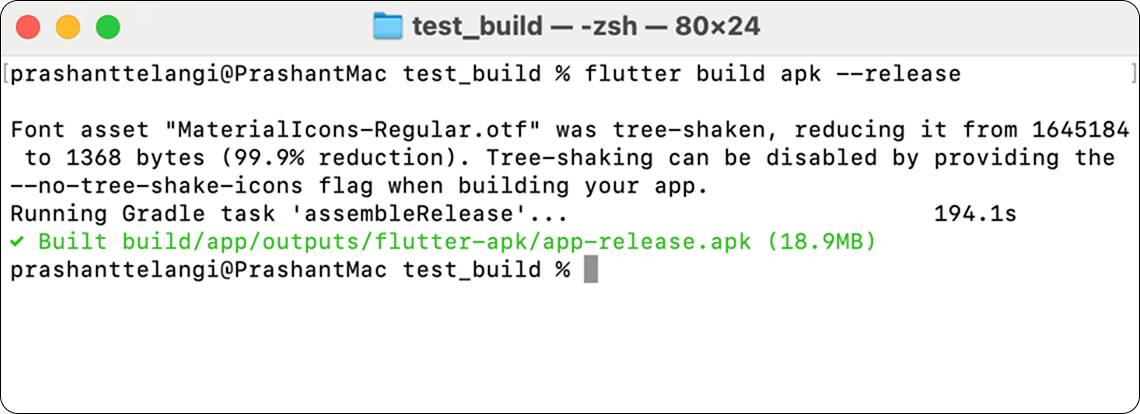
Step 2: Let’s develop a bash script to streamline the entire build creation process, including support for custom flavor builds.
#!/bin/bash
# Default values
FLAVOR="dev"
BUILD_NAME="1.0.0"
BUILD_NUMBER="1"
# Clean previous builds
echo "Cleaning previous builds..."
rm -rf build/
rm -rf .dart_tool/
rm -rf .flutter-plugins
rm -rf .flutter-plugins-dependencies
# Flutter clean and get dependencies
echo "Running flutter clean..."
flutter clean
echo "Getting dependencies..."
flutter pub get
...
# Build Android APK
echo "Building Android APK"
...
echo "Getting dependencies..."
flutter pub get
# Build command construction
BUILD_COMMAND="flutter build apk --flavor $FLAVOR -t lib/main_$FLAVOR.dart"
# Add version and build number if provided
if [ ! -z "$BUILD_NAME" ]; then
BUILD_COMMAND="$BUILD_COMMAND --build-name=$BUILD_NAME"
fi
if [ ! -z "$BUILD_NUMBER" ]; then
BUILD_COMMAND="$BUILD_COMMAND --build-number=$BUILD_NUMBER"
fi
# Execute build
echo "Building $FLAVOR flavor..."
echo "Executing: $BUILD_COMMAND"
eval $BUILD_COMMAND
# Check if build was successful
if [ $? -eq 0 ]; then
echo "Build completed successfully!"
APK_PATH="build/app/outputs/flutter-apk/app-$FLAVOR-release.apk"
echo "APK location: $APK_PATH"
else
echo "Build failed!"
exit 1
fi
Code language: PHP (php)
#Usage :
build_android_flutter.sh -f dev -v BUILD_NAME -b BUILD_NUMBER
Version Control:
- Accepts two optional parameters:
-v
for version name (e.g., “1.0.0”)-b
for build number (e.g., “1”)
Flavors Specific Arguments:
-f “$FLAVOR”:
- Specifies which flavor (variant) of the app to build
- Common flavors might be ‘dev’, ‘staging’, ‘prod’
-t “lib/main_$FLAVOR.dart”:
- Specifies the entry point file for the app
- Uses different main files for different flavors
- Example: main_dev.dart, main_prod.dart
Build Process:
- Cleans previous builds using
flutter clean
- Updates project dependencies with
flutter pub get
- Builds the APK file with the provided flavor, version and build number
Output:
- The final APK file is created in the
./build/app/outputs/flutter-apk/
directory - Detailed logs are displayed throughout the build process

Usage Example:
sh build_android_flutter.sh -f dev -v 1.0.0 -b 1
Code language: CSS (css)
This above will build your Android APK for flavor dev with version 1.0.0 and build number 1. If you don’t provide these parameters, the script will use the default values (flavor=”dev”, version=”1.0.0″, build=”1″).
Note: Copy the above bash script to your Flutter project directory and ensure it has the correct permissions. Grant the script read and write access by executing the following command in your terminal:
chmod +x build_android_flutter.sh
Code language: CSS (css)
Summing It Up
I hope you found this tutorial on Creating Android Builds for Flutter Apps with a Custom Build Script helpful. You can download the complete Flutter Android build script from here.

Author's Bio
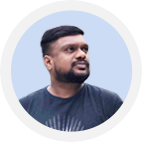
Prashant Telangi brings over 15 years of experience in Mobile Technology, He is currently serving as Head of Technology, Mobile at Mobisoft Infotech. With a proven history in IT and services, he is a skilled, passionate developer specializing in Mobile Applications. His strong engineering background underscores his commitment to crafting innovative solutions in the ever-evolving tech landscape.