In order to delete a call log entry in android device/ Emulator, first of all, we must know where the log information is stored
. займ 15000 рублей. Android stores the log information in its internal database as a content provider – “content://call_log/calls”.
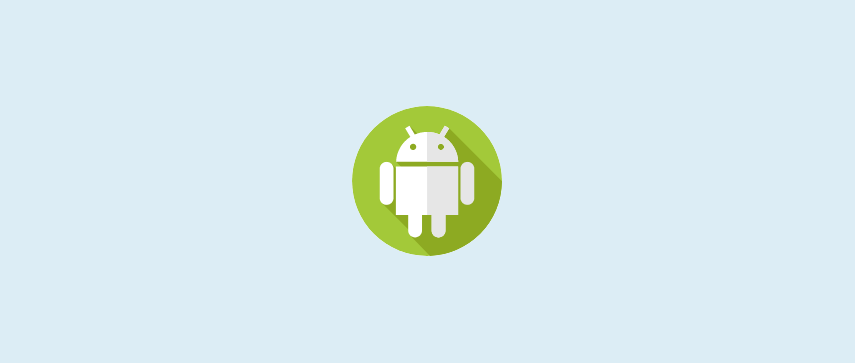
Call log Basics
A content provider is used to share data between multiple applications. In Android, a content provider is a specialized type of data store that exposes standardized ways to retrieve and manipulate the stored data.
The call log data is used by multiple applications and hence android stores it as a content provider. The Call Log provider contains information about placed and received calls.
To do:
- Get uri for call log content provider.
- Query the content provider.
- Search the row in result set(curser) .
- Delete the row from content provider.
URI
The call log content provider exposes a public URI “content://call_log/calls” for recent phone calls that uniquely identifies its data set.
Uri allCalls = Uri.parse(“content://call_log/calls”);
Querying a Content Provider
To access database we need to query the content provider by using the uri which the given content provider exposes.
Cursor c = managedQuery(allCalls, null, null, null, null);
Reading retrieved data for deleting particular log entry
Cursor c stores record set of results regarding the log details. So, now in order to delete any record single record, call ContentResolver.delete()
with the URI of a specific row.
To delete multiple rows, call ContentResolver.delete()
with the URI of the type of record to delete (for example, android.provider.CallLog.Calls.CONTENT_URI
) and an SQL WHERE
clause defining which rows to delete.
Here we will delete the row by comparing ‘NUMBER’ column with the number entered by user.
while(c.moveToNext())
{
// get particular number for which log entry is to be deleted.
String strNumber= etNumberForCall.getText().toString();
// make a selection clause.
String queryString= “NUMBER='” + strNumber + “‘”;
Log.v(“Number”, queryString);
CallLogActivity.this.getContentResolver().delete(UriCalls, queryString, null);
}
Screen Shorts:
DeleteScreen
ResultScreen
Following is complete source code:
[java] package com.mobisoftinfotech; import android.app.Activity; import android.database.Cursor; import android.net.Uri; import android.os.Bundle; import android.util.Log; import android.view.View; import android.view.View.OnClickListener; import android.widget.Button; import android.widget.EditText; importandroid.widget.Toast; /** * @author Mohd. Akram */ public class CallLogActivity extends Activity { /** Called when the activity is first created. */ EditText etNumberForCall; Button btnDeleteNumberFromCallLog; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.main); etNumberForCall=(EditText)findViewById(R.id.EditText01); btnDeleteNumberFromCallLog=(Button)findViewById(R.id.Button01); btnDeleteNumberFromCallLog.setOnClickListener(new OnClickListener() { @Override public void onClick(View v) { //TODO Auto-generated method stub String strUriCalls="content://call_log/calls"; Uri UriCalls = Uri.parse(strUriCalls); Cursor c = CallLogActivity.this.getContentResolver().query(UriCalls, null, null, null, null); if (c.getCount()<=0) { Toast.makeText(getApplicationContext(), "Call log empty",Toast.LENGTH_SHORT).show(); etNumberForCall.setText(""); } while (c.moveToNext()) { String strNumber= etNumberForCall.getText().toString(); String queryString= "NUMBER='" + strNumber + "'"; Log.v("Number", queryString); int i=CallLogActivity.this.getContentResolver().delete(UriCalls, queryString, null); etNumberForCall.setText(""); if(i>=1) { Toast.makeText(getApplicationContext(), "Number deleted", Toast.LENGTH_SHORT).show(); } else { Toast.makeText(getApplicationContext(), "No such number in call logs", Toast.LENGTH_SHORT).show(); } } } }); } } [/java]
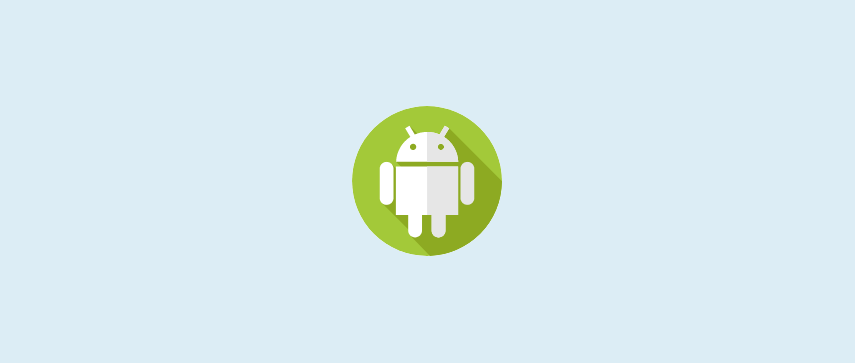