Instagram is one of the top applications to share pictures, videos with your friends and family. It allows you edit photos taken and add some effects. Android or iOS application can retrieve data from Instagram. Also can share photos from your own application over Instagram.
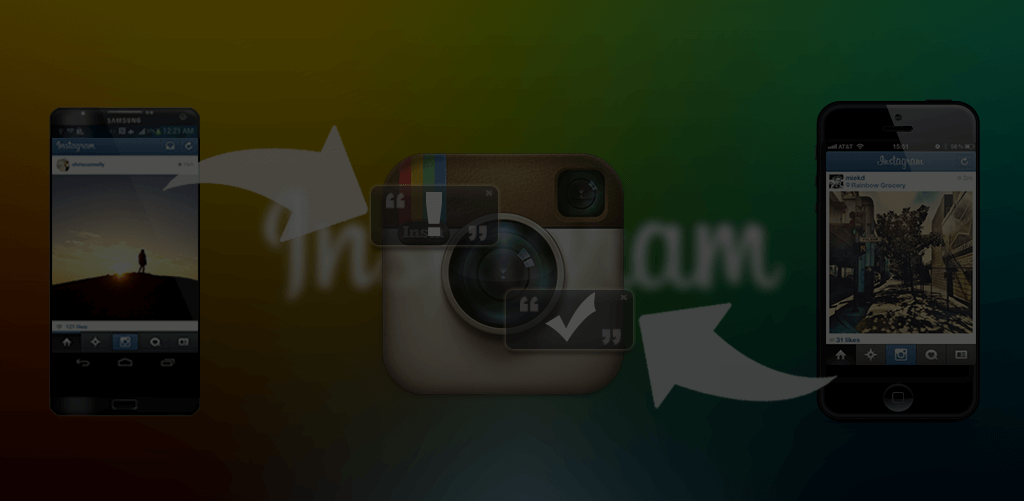
Instagram provides few APIs to fetch data from Instagram. Also some APIs to post comments and likes over Instagram. All the APIs are based on OAuth 2.0 protocol.
Link to Instagram APIs enpoints documentation :- http://instagram.com/developer/endpoints/
Tool to test Instagram APIs :- http://instagram.com/developer/api-console/
However if a developer wants to share photos from his/her own application Instagram, Instagram does not provide any direct API for that. Iphone hooks is a feature provided by Instagram developer community to share photo from iOS application. Instagram does not provide any support to share photos from Android application. Still sharing photos over Instagram is possible via android intent. I would like to share code snippet to share a photo from both Android and iOS along with its limitations.
Sharing photo from iOS application over Instagram
Following code snippet shares a photo over Instagram. Caption can be set programmatically for image to be shared. This needs Instagram app installed over your iOS device. If Instagram app is not already installed then it will show alert message saying “To share image please install Instagram.”. Photo sharing is done using Document Interaction API which open your photo in Instagram.
-(void)sharePhotoToInstagram { NSURL *instagramURL = [NSURL URLWithString:@"instagram://"]; if ([[UIApplication sharedApplication] canOpenURL:instagramURL]) { CGRect rect = CGRectMake(0,0,0,0); NSString *jpgPath=[NSHomeDirectory() stringByAppendingPathComponent:@"Documents/Home_1_instgram.igo"]; UIImage *imageN = [UIImage imageWithContentsOfFile:[NSString stringWithFormat:@"%@", jpgPath]]; [UIImagePNGRepresentation(img1) writeToFile:jpgPath atomically:YES]; NSURL *igImageHookFile = [[NSURL alloc] initWithString:[[NSString alloc] initWithFormat:@"file://%@",jpgPath]]; self.docFile.UTI = @"com.instagram.exclusivegram"; self.docFile = [self setupControllerWithURL:igImageHookFile usingDelegate:self]; self.docFile.annotation = [NSDictionary dictionaryWithObject:[NSString stringWithFormat:@"Amazing Video Please watch\n%@",videoshareUrl] forKey:@"InstagramCaption"]; [self.docFile presentOpenInMenuFromRect: rect inView: self.view animated: YES ]; } else { UIAlertView *alert=[[UIAlertView alloc] initWithTitle:@"Message" message:@"Instagram not installed in this device!\nTo share image please install instagram." delegate:nil cancelButtonTitle:nil otherButtonTitles:@"ok", nil]; [alert show]; } }
Sharing photo from Android application over Instagram
Instagram does not provide any direct way to share photos from Android application on Instagram. It is possible via an intent if Instagram application is installed on device. Instagram application has package name “com.instagram.android”. So intent can be called in following way.
private void shareOverstagram(Uri uri) { Intent shareIntent = new Intent(android.content.Intent.ACTION_SEND); shareIntent.setType("image/*"); shareIntent.putExtra(Intent.EXTRA_STREAM, uri); // set uri shareIntent.setPackage("com.instagram.android"); startActivity(shareIntent); }
Above function needs Uri which you want to share over Instagram. This Uri can be of any photo or image from your device SD card. Before calling above function one has to write code to check Instagram is already installed or not.
private boolean appInstalledOrNot() { boolean app_installed = false; try { ApplicationInfo info = activity.getPackageManager().getApplicationInfo("com.instagram.android", 0); app_installed = true; } catch (PackageManager.NameNotFoundException e) { app_installed = false; } return app_installed; }
Major limitation of Android as compared to iOS :-
Major difference between Android and iOS implementation to share photo over Instagram is, one cannot set caption for photo to be shared programmatically in Android. In case of iOS it is possible to set caption for photo through code. Android intent does not recognize any param set in “putExtra” of intent.
I hope Instagram comes up with something soon which allows us to share photo with caption from Android application as well like iOS.